Browse Course Material
Course info, instructors.
- Daniel Weller
- Sharat Chikkerur

Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Practical programming in c, assignments.

You are leaving MIT OpenCourseWare
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
C Exercises - Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
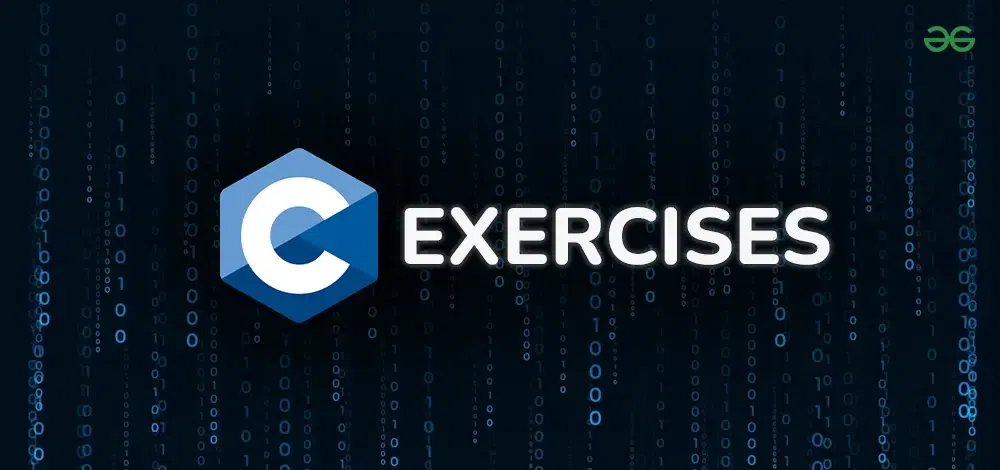
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
Also, Once you've covered basic C exercises, the GeeksforGeeks Practice Platform is a great place to take on more advanced C coding problems and improve your understanding.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print "Hello World!" on the Console.
In this problem, you have to write a simple program that prints "Hello World!" on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
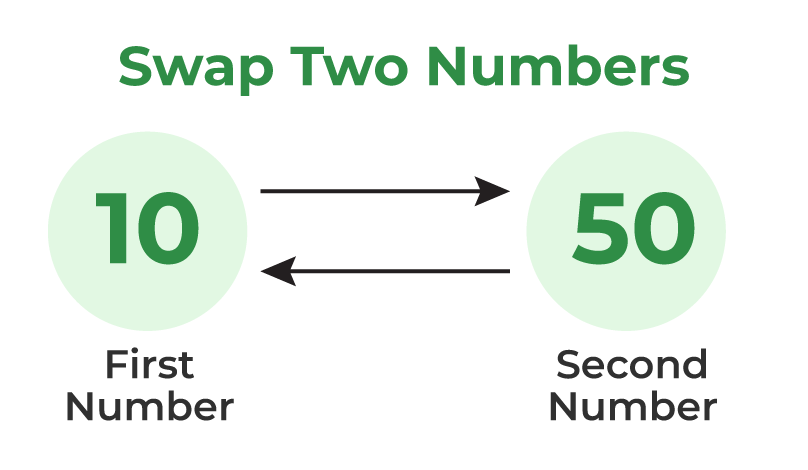
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
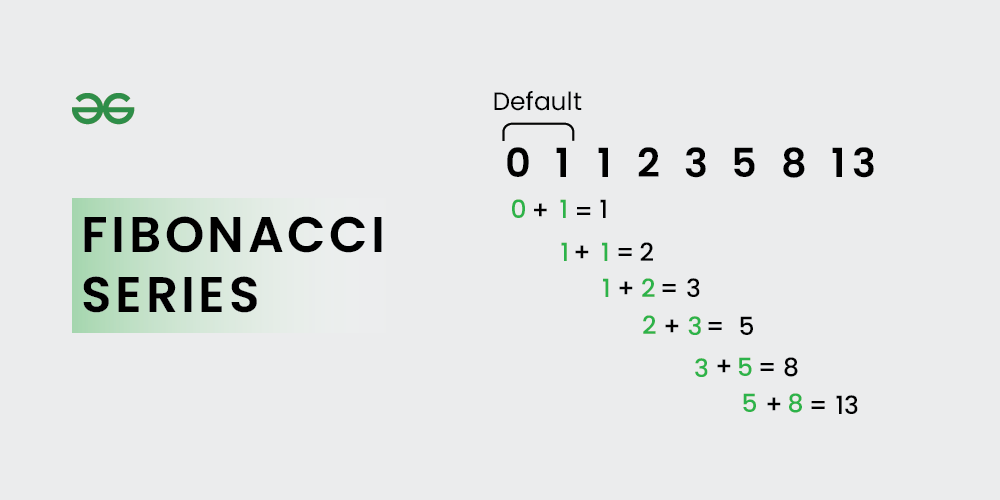
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
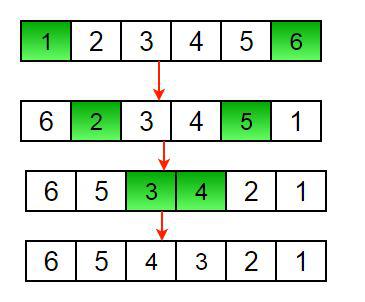
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print 'element is not present in array '.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print 'str is a palindrome' else print 'str is not a palindrome'. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
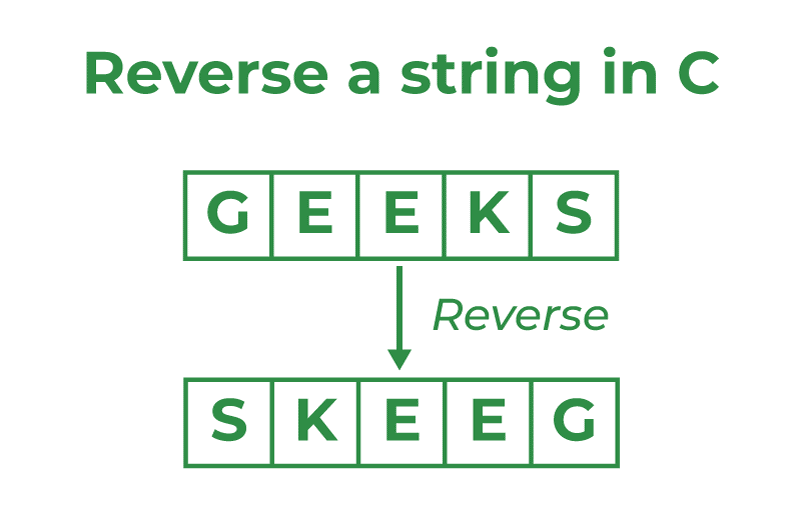
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.

Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
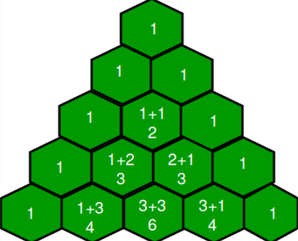
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message "Cannot open file: file_name" and terminate the program else print "Content copied to file_name"
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student's record. Initialize the records with sample data having data members' Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Similar Reads
- C Exercises - Practice Questions with Solutions for C Programming The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more. So, Keep it U 12 min read
- Commonly Asked C Programming Interview Questions | Set 3 Q.1 Write down the smallest executable code? Ans. main is necessary for executing the code. Code is [GFGTABS] C void main() { } [/GFGTABS]Output Q.2 What are entry control and exit control loops? Ans. C support only 2 loops: Entry Control: This loop is categorized in 2 part a. while loop b. for loop 6 min read
- Interesting Facts in C Programming | Set 2 Below are some more interesting facts about C programming: 1. Macros can have unbalanced braces: When we use #define for a constant, the preprocessor produces a C program where the defined constant is searched and matching tokens are replaced with the given expression. Example: [GFGTABS] C #include 2 min read
- C/C++ Program for String Search C/C++ Program for Naive Pattern SearchingC/C++ Program for KMP AlgorithmC/C++ Program for Rabin-Karp AlgorithmC/C++ Program for A Naive Pattern Searching QuestionC/C++ Program for Finite AutomataC/C++ Program for Efficient Construction of Finite AutomataC/C++ Program for Boyer Moore Algorithm – Bad 1 min read
- How to Write a Command Line Program in C? In C, we can provide arguments to a program while running it from the command line interface. These arguments are called command-line arguments. In this article, we will learn how to write a command line program in C. How to Write a Command Line Program in C? Command line arguments are passed to the 2 min read
- C Multiple Choice Questions C is the most popular programming language developed by Dennis Ritchie at the Bell Laboratories in 1972 to develop the UNIX operating systems. It is a general-purpose and procedural programming language. It is faster than the languages like Java and Python. C is very versatile it can be used in both 4 min read
- C/C++ Mathematical Programs Mathematical Algorithms in programming are the specialized method to solve arithmetic problems such as finding roots, GCD, etc. Most of us are familiar with the conventional methods and concepts used to solve such problems but they may not be the best choice in programming, such as the best choice f 2 min read
- C/C++ Divide and Conquer Programs The divide and conquer is an algorithmic approach to solve problems by dividing them into smaller sub-problems, solving them, and then constructing the complete solution using the solution of smaller sub-problems. This approach uses the recursion to divide the problem into smaller subproblems and it 2 min read
- Matrix C/C++ Programs C Program to check if two given matrices are identicalC program to find transpose of a matrixC program for subtraction of matricesC program for addition of two matricesC program to multiply two matricesC/C++ Program for Print a given matrix in spiral formC/C++ Program for A Boolean Matrix QuestionC/ 1 min read
- Getting started with C C language is a popular programming language that was developed in 1970 by Dennis Ritchie at Bell Labs. The C programming language was developed primarily to build the UNIX operating system. It is widely used because it is simple, powerful, efficient, and portable. Features of C Programming Language 5 min read
- Examination Management System in C Problem Statement: Write C program to build a Software for Examination Management System that can perform the following operations: Add/Delete the Details of the StudentsAttendance Monitoring of the studentsSet/Edit Eligibility criteria for examsCheck Eligible Students for ExamsPrint all the records 9 min read
- C File Handling Programs C Program to list all files and sub-directories in a directory C Program to count number of lines in a file C Program to print contents of file C Program to copy contents of one file to another file C Program to merge contents of two files into a third file C program to delete a file 1 min read
- How to Read From a File in C? File handing in C is the process in which we create, open, read, write, and close operations on a file. C language provides different functions such as fopen(), fwrite(), fread(), fseek(), fprintf(), etc. to perform input, output, and many different C file operations in our program. In this article, 2 min read
- Misc C Programs C Program to print environment variablesC Program to Swap two NumbersC program swap two numbers without using a temporary variableC Program to check if a given year is leap yearC Program to sum the digits of a given number in single statement?C program to print numbers from 1 to 100 without using lo 1 min read
- C Programs To learn anything effectively, practicing and solving problems is essential. To help you master C programming, we have compiled over 100 C programming examples across various categories, including basic C programs, Fibonacci series, strings, arrays, base conversions, pattern printing, pointers, and 9 min read
- C++ Exercises - C++ Practice Set with Solutions Do you want to improve your command on C++ language? Explore our vast library of C++ exercise questions, which are specifically designed for beginners as well as for advanced programmers. We provide a large selection of coding exercises that cover every important topic, including classes, objects, a 15+ min read
- C Programming Interview Questions (2024) At Bell Labs, Dennis Ritchie developed the C programming language between 1971 and 1973. C is a mid-level structured-oriented programming and general-purpose programming. It is one of the oldest and most popular programming languages. There are many applications in which C programming language is us 15+ min read
- Commonly Asked C Programming Interview Questions | Set 2 This post is second set of Commonly Asked C Programming Interview Questions | Set 1What are main characteristics of C language? C is a procedural language. The main features of C language include low-level access to memory, simple set of keywords, and clean style. These features make it suitable for 3 min read
- Facts and Question related to Style of writing programs in C/C++ Here are some questions related to Style of writing C programs: Question-1: Why i++ executes faster than i + 1 ? Answer-1: The expression i++ requires a single machine instruction such as INR to carry out the increment operation whereas, i + 1 requires more instructions to carry out this operation. 2 min read
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −

- C All Exercises & Assignments
Write a C program to check whether a number is even or odd
Description:
Write a C program to check whether a number is even or odd.
Note: Even number is divided by 2 and give the remainder 0 but odd number is not divisible by 2 for eg. 4 is divisible by 2 and 9 is not divisible by 2.
Conditions:
- Create a variable with name of number.
- Take value from user for number variable.
Enter the Number=9 Number is Odd.
Write a C program to swap value of two variables using the third variable.
You need to create a C program to swap values of two variables using the third variable.
You can use a temp variable as a blank variable to swap the value of x and y.
- Take three variables for eg. x, y and temp.
- Swap the value of x and y variable.
Write a C program to check whether a user is eligible to vote or not.
You need to create a C program to check whether a user is eligible to vote or not.
- Minimum age required for voting is 18.
- You can use decision making statement.
Enter your age=28 User is eligible to vote
Write a C program to check whether an alphabet is Vowel or Consonant
You need to create a C program to check whether an alphabet is Vowel or Consonant.
- Create a character type variable with name of alphabet and take the value from the user.
- You can use conditional statements.
Enter an alphabet: O O is a vowel.
Write a C program to find the maximum number between three numbers
You need to write a C program to find the maximum number between three numbers.
- Create three variables in c with name of number1, number2 and number3
- Find out the maximum number using the nested if-else statement
Enter three numbers: 10 20 30 Number3 is max with value of 30
Write a C program to check whether number is positive, negative or zero
You need to write a C program to check whether number is positive, negative or zero
- Create variable with name of number and the value will taken by user or console
- Create this c program code using else if ladder statement
Enter a number : 10 10 is positive
Write a C program to calculate Electricity bill.
You need to write a C program to calculate electricity bill using if-else statements.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements.
Enter the units consumed=278.90 Electricity Bill=1282.84 Rupees
Write a C program to print 1 to 10 numbers using the while loop
You need to create a C program to print 1 to 10 numbers using the while loop
- Create a variable for the loop iteration
- Use increment operator in while loop
1 2 3 4 5 6 7 8 9 10
- C Exercises Categories
- C Top Exercises
- C Decision Making

C Programming Exercises With Solutions (PDF)
Here you will get C Programming Exercises With Solutions.
Here I am going to provide you a document of these, which you can download and make changes according to your own.

Download C Programming Practical Assignments Questions
Download Now
Download C Programming Exercises With Solutions PDF (2020)
Download c programming exercises with solutions pdf (2021), download 100+ c programming exercises pdf.
If you face any problem in downloading C Programming Practical Assignments Questions and Solutions PDFs, then tell us in the comment below and you will definitely share this post with your friends so that they can also be of some help.
Also Read -:
- Database Management System Lab Assignment Exercise Question and There Solutions
- C++ Lab Exercises And Solutions (PDF) – Master Programming
- Web Technology Lab Assignment Question And Solutions
- Pc Software Practical Assignment Question And Solutions
- Operating System with Linux Lab Assignment Question And Solutions (PDF)
Assignment Statement (=) in C Language
Assignment Statement in C language is a statement that assigns or set a value to a variable during program execution. Assignement statements in programming allows the programmer to change or set the value stored in variable using Assignment(=) Operator. The process of assigning the value to a variable using the assignment(=) operator is known as an assignment statement in C. Assignment(=) Operator Assigns The value or value in a variable on right hand side to the variable on the left hand side. The data type of the variable on right hand side should match to the data type of variable or constant or expression on right hand side. C Language has different (types) ways to assigns values to variable, we will learn from the diagram given below.
Syntax :1.Basic Assignment statement
Data Type Variable_name = variable/ constant /expression; The Variable_name is assigned the values in variable or constants or expression. The data type of the variable/ constant/expression on right hand side should match to the left hand side variable Variable_name with a few exceptions where automatic type conversions are possible.
Naming Rules or conventions for Assignment Statement
Programmer need to follow some Rules while writing the Assignment Statements in C program: 1. Variable names should not begin or start with number. Variable name can a letter, underscore, non-number any character like alphabet,underscore. 2. A new value assigned to an existing variable will overwrite the previous value and assign the new value to the variable. 3. The Data type defined and the variable value must match. 4.All the statements declaration must end with a semi-colon. (;) 5. The name of variable must be meaningful and clearly describe the purpose of variable name. 6.Duplicate name of variable is not allowed i.e the name once defined can only be used once in the program. programmer cannot redefine it to store other types of value.

Example 1: C program to illustrates the use of Simple Assignment statement .
/* e.g. C program to illustrate the use of simple Assignment statement or basic of assignment statements */ #include<stdio.h> int main() { int a,b,c; float avg; a = 9 ; c = 10 ; b = c ; printf("\n a=%d",a); printf("\n b=%d",b); printf("\n c=%d",c); b = c+3; printf("\n Value of b after b=c+3-->%d",b); avg = (b+c) / 2.0; printf("\n Value of avg=%.2f",avg); a = b && c; printf("\n a=b&&c--->%d",a); a = (b+c) && (b <c); printf("\n a=(b+c) && (b <c)--->%d",a); return(0); } Output: a=9 b=10 c=10 Value of b after b=c+3-->13 Value of avg=11.50 a=b&&c--->1 a=(b+c) && (b <c)--->0
Program Explanation: 1. In the above program a,b,c is declared as integer variable to store the numbers where as avg is declared as float. int a,b,c; float avg; 2. a = 9 ; c = 10 ; b = c ; variable a is assigned value 9. variable c is assigned value 10. and b is assigned the value of c. Here value of b is 10 .i.e. b=10 3. printf("\n a=%d",a); printf("\n b=%d",b); printf("\n c=%d",c); The above statements displays the integer values of variable a,b,c a=9 b=10 c=10 4. b = c+3; printf("\n Value of b after b=c+3-->%d",b); here 3 is added in c(value of vaiable c is 10) it becomes 13 and value 13 is assigned to left hand side variable b , here b is 13 i.e. b=13. the printf() displays output " Value of b after b=c+3--->13". 5. avg = (b+c) / 2.0; printf("\n Value of avg=%.2f",avg); After execution of a=(b+c)/2.0 the value of a is 11.50 so the output shown by printf() is "Value of avg=11.50". 6. a = b && c; printf("\n a=b&&c--->%d",a); Logical anding operation is performed on the values in the variables a and b. The value of c=10 and the value of b=13. After succesful execution of this statement value of variable a is 1 or 'true'. Any value in a variable except 'zero' (0 i.e false) is considered 'true' or 1. The variable a is assigned to the integer value 1 which is true. so a=1 && 1 is a=1 or a=true && true is a=true or a=1. printf("\n a=b&&c--->%d",a); displays output a=b&&c---->1 7. a = (b+c) && (b <c); printf("\n a=(b+c) && (b <c)--->%d",a); here expression (b+c) is true(1) and (b <c) is false(0). so the entire expression evaluates to false(0) and the value is assigned to the variable a i.e a=0. the printf() display the message " a=(b+c) && (b <c)--->0 "
2.Compound Assignment: As we learn in above section the simple assignment statement is used to assign values in right hand side variable to left hand side variable using = operator. A compound assignment operator has a shorter syntax to assign the result. A compound assignment operator or statements are used to do mathematical operation in shortcut. Two operands needs to perform the compound assignment operations. The operation is performed on the two operands before the result is assigned to the first operand. compound assignment operator are binary operators that modify the variable to their left hand side using the value or variable to their right.
Syntax: expression1+= expression2; These type of expression can also be written in expanded form, that is expression1=expression1+expression2;
+= Operator is called compound assignment operator. C Language provides the following list of compound Assignment Operators. 1. += plus equal to += is Addition and Assignment operators. It add the value of the variable1 and variable2 and assigns the result to variable1. e.g. X+=Y In the above expression the addition of values in X and Y is performd and assigns result to X. The expression X+=Y is same as X=X+Y 2. -= minus equal to -= is Subtraction and Assignment operators. It Subtract the value of the variable2 from variable1 and assigns the result to variable1. e.g. X-=Y In the above expression the subtraction is performd,the value of X is subtracted from the value in X and assigns result to X. The expression X-=Y is same as X=X-Y 3. *= Multiplication equal to *= is Multiplication and Assignment operators. It Multiply the value of the variable1 and variable2 and assigns the result to variable1. e.g. X*=Y In the above expression the Multiplication is performd,the value of X is Multiplyed to the value in Y and assigns result to X. The expression X*=Y is same as X=X*Y 4. /= Division equal to *= is Division and Assignment operators. It Divides the value of the variable1 byvariable2 and assigns the result to variable1. e.g. X/=Y In the above expression the Division is performd,the value of X is Divided by the value in Y and assigns result to X. The expression X/=Y is same as X=X/Y 5. %= Modulus equal to %= is Modulus and Assignment operators. It Divides the value of the variable1 byvariable2 and assigns the remainder to variable1. e.g. X%=Y In the above expression the Division is performd,the value of X is Divided by the value in Y and assigns remainder to X. The expression X%=Y is same as X=X%Y 6. &= Bitwise and equal to &= is Bitwise AND and Assignment operators. It performs the bitwise AND with variable1 and variable2 and assigns the result to variable1. e.g. X&=Y In the above expression the bitwise anding operation is performd, after executing the X&=Y expression the result is assigned to X. The expression X&=Y is same as X=X&Y 7. |= Bitwise OR equal to != is Bitwise OR Assignment operators. It performs the bitwise OR with variable1 and variable2 and assigns the result to variable1. e.g. X|=Y In the above expression the bitwise OR operation is performd, after executing the X|=Y expression the result is assigned to X. The expression X|=Y is same as X=X|Y 8. ^= Bitwise XOR equal to ^= is Bitwise XOR Assignment operators. It performs the bitwise XOR with variable1 and variable2 and assigns the result to variable1. e.g. X^=Y In the above expression the bitwise XOR operation is performd, after executing the X^=Y expression the result is assigned to X. The expression X^=Y is same as X=X^Y. 9. Bitwise left shift and equal to e.g. X In the above expression the bitwise left shift operation is performd, after executing the X The expression X 10. >>= Bitwise right shift and equal to >>= is Bitwise Right Shift and Assignment operators. It performs the bitwise Right shift with variable1 and assigns the result to variable1. e.g. X>>=Y In the above expression the bitwise right shift operation is performd, after executing the X>>=Y expression the result is assigned to X. The expression X>>=Y is same as X=X>>Y Lets Learn and practice The Assigment operator in detail using the following C program.
2. Assignment Operator complete C Program.
#include <stdio.h> int main() { /* Simple Assignment*/ int x,i; int y,j; float c=30.0; float d=5.0; /*Nested or Multiple Assignment */ x = i = 5; y = j = 3; /*Compound Assignment*/ x += y; printf("After Add and Assign :%d \n",x); i -= j; printf("After Subtract and Assign :%d \n",i); x *= y; printf("After Multiple and Assign :%d \n",x); c /= d; printf("After Divide and Assign :%f \n",c); j %= i; printf("After Modulo and Assign :%d \n",j); j &= i; printf("After Bitwise And and Assign :%d \n",j); j |= i; printf("After Bitwise OR and Assign :%d \n",j); x ^= y; printf("After Bitwise XOR and Assign :%d \n",a); x printf ("After Bitwise Left Shift and Assign :%d \n",a); x >>= 3; printf ("After Bitwise Right Shift and Assign :%d \n",a); return(0); }
Output: After Add and Assign :8 After Subtract and Assign :2 After Multiple and Assign :24 After Divide and Assign :6.000000 After Modulo and Assign :1 After Bitwise And and Assign :0 After Bitwise OR and Assign :2 After Bitwise XOR and Assign :27 After Bitwise Left Shift and Assign :108 After Bitwise Right Shift and Assign :13
/** * C program to check leap year using conditional operator ? */ #include <stdio.h> int main() { int year; /* * Input the year from user */ printf("Enter any year: "); scanf("%d", &year); /* * If year%4==0 and year%100==0 then * print leap year * else if year%400==0 then * print leap year * else * print common year */ (year%4==0 && year%100!=0) ? printf("LEAP YEAR") : (year%400 ==0 ) ? printf("LEAP YEAR") : printf("COMMON YEAR"); return 0; } Output: Enter any year 2004 LEAP YEAR
/** * C program to check leap year using conditional operator ? */ #include <stdio.h> int main() { int year; /* * Input the year from user */ printf("Enter any year: "); scanf("%d", &year); /* If year%4==0 and year%100==0 then print leap year else if year%400==0 then print leap year else print common year */ (year%4==0 && year%100!=0) ? printf("LEAP YEAR") : (year%400 ==0 ) ? printf("LEAP YEAR") : printf("COMMON YEAR"); return 0; } Output: Enter any year 2004 LEAP YEAR
Previous Topic:-->> Constant and Literals in C || Next topic:-->> Input/Output in C.
Get in touch

IMAGES
COMMENTS
Mar 20, 2024 · At Bell Labs, Dennis Ritchie developed the C programming language between 1971 and 1973. C is a mid-level structured-oriented programming and general-purpose programming. It is one of the oldest and most popular programming languages. There are many applications in which C programming language is us
3 days ago · C is a general-purpose, imperative computer programming language, supporting structured programming, lexical variable scope and recursion, while a static type system prevents many unintended operations. C was originally developed by Dennis Ritchie between 1969 and 1973 at Bell Labs.
(This ZIP file contains: 1 .txt file and 2 .c files.) 6b Function pointers, hash table (This ZIP file contains: 1 .txt file and 2 .c files.) 7 Using and creating libraries, B-trees and priority queues (This ZIP file contains: 2 .c files and 1 .db file.)
Sep 23, 2024 · C language is a popular programming language that was developed in 1970 by Dennis Ritchie at Bell Labs. The C programming language was developed primarily to build the UNIX operating system. It is widely used because it is simple, powerful, efficient, and portable. Features of C Programming Language
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression. The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol ...
Sep 21, 2024 · In C programming, assignment operators are used to assign values to variables. The simple assignment operator is =. C also supports shorthand assignment operators that combine an operation with assignment, making the code more concise. Key Topics: Simple Assignment Operator; Shorthand Addition Assignment (+=) Shorthand Subtraction Assignment ...
Write a C program to find the maximum number between three numbers . Description: You need to write a C program to find the maximum number between three numbers. Conditions: Create three variables in c with name of number1, number2 and number3; Find out the maximum number using the nested if-else statement
Jan 6, 2024 · Download 100+ C Programming Exercises PDF . Download Now . If you face any problem in downloading C Programming Practical Assignments Questions and Solutions PDFs, then tell us in the comment below and you will definitely share this post with your friends so that they can also be of some help. Also Read -:
1.Simple Assignment or Basic Form: Simple Assignment or Basic form is one of the common forms of Assignment Statements in C program. Simple Assignment allow the programmer to declare,define and initialize and assign a value in the same statement to a variable. This form is generally used when the programmer want to use the Variable a few times.
The Assignment operators in C are some of the Programming operators that are useful for assigning the values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: int i = 10; The below table displays all the assignment operators present in C Programming with an example.