Convert Text to Acronyms in Python – A Complete Guide

An acronym is a shortened version of a long phrase, for instance, we have NLP for natural language processing . They are widely used in the world of programming, data science, and even in our daily life. They help simplify lengthy phrases or names into short, easily recognizable terms. This not only saves space but also makes reading more efficient and easy to comprehend.
In this comprehensive Python tutorial, we will demonstrate how to create an acronym generator using Python.
While spelling out the whole word takes longer, saying or writing the first initial of each word or an abbreviated form of the full word takes less time. In programming, as well as in everyday speech, employing acronyms and abbreviations can make communication more efficient.
Python, owing to its simplicity and effective string-handling capabilities, is an ideal language for creating an acronym generator. Python’s prowess in natural language processing tasks further justifies its use for this application. With Python, we can efficiently break down sentences and extract required components.
Also read: Crypto Price Prediction with Python

Converting Text to Acronyms – Acronym Generator in Python
To implement acronyms, our aim is to generate a short form of a word from a given sentence. In this tutorial, our aim is to demonstrate how to create an acronym generator in Python. The same can be done by splitting and indexing to get the first word and then combining it.
Within the Python ecosystem, we leverage several built-in methods for this task. The split() function is used to break down the sentence into individual words. The upper() function assists in maintaining the convention of having acronyms in uppercase. This section gives an insight into how Python handles strings and utilizes lists and for loops
Look at the code mentioned below and then we will go inside the code line by line.
- Line 1 takes the input of the Phrase which needs to be converted into an acronym with the help of the input function.
- Line 2 will convert the sentence into a list of words with the help of the split function which will by default split the sentence on the basis of whitespaces.
- Line 3 is initializing the final acronym with an empty string which will be changed later on.
- From Line 4 and Line 5 , we have a loop that will add the first letter of each word as a character in the final acronym.
- The acronyms always include uppercase letters hence, each first letter in every word is converted to uppercase regardless of their previous case with the help of the upper function.
- From Line 7 to Line 9 will display the final acronym that has been created with the help of loops and print statements.
This acronym generator has potential applications in data preprocessing for Natural Language Processing (NLP) tasks, or in automating the process of acronym creation in large documents or articles. Furthermore, the code can be improved to handle punctuation and mixed case phrases, extending its utility
Output examples of creating acronym using Python
The images below display some sample outputs after executing the code mentioned in the previous section.

By now, you should have a solid understanding of how to create an acronym generator in Python. This practical tool can simplify long phrases into manageable terms. As you continue to grow in your Python programming journey, what other applications can you envision developing to make everyday tasks easier?
Want to learn more? Check out the tutorials mentioned below:
- Find Number of Possible Strings With No Consecutive 1s
- How to convert a dictionary to a string in Python?
- Convert a Tuple to a String in Python [Step-by-Step]
- String Formatting in Python – A Quick Overview
- Creating acronyms in Python – Stack Overflow
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python – Create acronyms from words
Given a string, the task is to write a Python program to extract the acronym from that string.
Input: Computer Science Engineering Output: CSE Input: geeks for geeks Output: GFG Input: Uttar pradesh Output: UP
Approach 1:
The following steps are required:
- Take input as a string.
- Add the first letter of string to output.
- Iterate over the full string and add every next letter to space to output.
- Change the output to uppercase(required acronym).
Time Complexity: O(n) -> looping once through string of length n.
Space Complexity: O(n) -> as space is required for storing individual characters of a string of length n.
Approach 2:
- Split the words.
- Iterate over words and add the first letter to output.
Time Complexity: O(n) -> looping once through an array of length n.
Space Complexity: O(n) -> as space is required for storing individual characters of a string and an array of length n.
Approach 3: Using regex and reduce()
Here’s one approach using regular expressions (regex) to extract the first letter of each word and reduce function to concatenate them:
Auxiliary Space: O(n) -> as space is required for storing individual characters of a string and an array of length n.
Similar Reads
- Python Programs
- Python string-programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Acronym in Python
Suppose we have a string s that is representing a phrase, we have to find its acronym. The acronyms should be capitalized and should not include the word "and".
So, if the input is like "Indian Space Research Organisation", then the output will be ISRO
To solve this, we will follow these steps −
tokens:= each word of s as an array
string:= blank string
for each word in tokens, do
if word is not "and", then
string := string concatenate first letter of word
return convert string into uppercase string
Let us see the following implementation to get better understanding −
Live Demo

- Related Articles
- How to include an acronym in HTML?
- Returning acronym based on a string in JavaScript
- What is the difference between an acronym and abbr tags?
- Counters in Python?
- sort() in Python
- string.octdigits in Python
- string.punctuation in Python
- string.whitespace in Python
- trunc() in Python
- Heaters in Python
- Quine in Python
- Underscore(_) in Python
- Multiprocessing In Python
- Keywords in Python
- ChainMap in Python
Kickstart Your Career
Get certified by completing the course

Acronyms Using Python
- January 13, 2021
- Machine Learning
An acronym is a short form of a word created by long words or phrases such as NLP for natural language processing. In this article, I will walk you through how to write a program to create acronyms using Python.
Create Acronyms using Python
To create acronyms using Python, you need to write a python program that generates a short form of a word from a given sentence. You can do this by splitting and indexing to get the first word and then combine it. Let’s see how to create an acronym using Python:
Enter a Phrase: Artificial Intelligence AI
In the above code, I am first taking a string user input, then I am using the split() function in Python for splitting the sentence. Then I declared a new variable ‘a’ to store the acronym of a phrase.
Also, Read – 100+ Machine Learning Projects Solved and Explained.
Then at the end, I am running a for loop over the variable ‘text’ which represents the split of user input. While running the for loop we are storing the index value of str[0] of every word after a split and turning it into an uppercase format by using the upper() function.
This is a great python program to test your logical skills. These types of programs contribute a lot to your coding interviews. So you should keep trying such programs to develop a good understanding of creating algorithms to perform well in your coding interviews.
I hope you liked this article on how to create acronyms with the Python programming language. Feel free to ask your valuable questions in the comments section below.
Aman Kharwal
Data Strategist at Statso. My aim is to decode data science for the real world in the most simple words.
Recommended For You

From ML Algorithms to GenAI & LLMs: Book Overview
- October 26, 2024

Python Problems for Coding Interviews
- August 23, 2024

Python Modules for Data Science You Should Know
- May 21, 2024

How Much Python is Required to Learn Data Science?
- January 3, 2024

13 Comments
Not sure if your code is correct or the full code.
The code is correct, open it on a desktop site you will get to see the complete code
Hi Aman, I can’t find how to thank u, but it’s so kind of you, thank u so much dude, I wish you a good luck
Thank you so much, keep visiting
Hello Sir, actually i starting my carrier in python as a beginner .. can you help me for python technical round coding questions .. it’s very tough for finding it.. if you share your blog or other link ….i will be thankfully of you.. and your contribution in knowledge share is amazing …salute sir
Here is an article based on the most popular coding interview questions solved using Python.
thank you so much
keep visiting
Thanks Aman for the great work. As a beginner ,i can’t think of a better place than this one.Thanks again
keep visiting 🤝
Thanks man. For such a detailed exploration of the projects. Very useful to me
keep visiting 😀🤝
hi Aman sir , I am just now started your projects ..it helps me in interviews…thank you so much
Leave a Reply Cancel reply
Discover more from thecleverprogrammer.
Subscribe now to keep reading and get access to the full archive.
Type your email…
Continue reading
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- How it works
- Homework answers
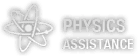
Answer to Question #228703 in Python for srikanth
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Average of Given Numbers You are given space-separated integers as input. Write a program to print
- 2. Largest Number in the List You are given space-separated integers as input. Write a program to prin
- 3. Replacing Characters of Sentence You are given a string S. Write a program to replace each letter
- 4. Diamond Given an integer value N, write a program to print a number diamond of 2*N -1 rows as sho
- 5. Diamond Crystal Given an integer value N, write a program to print a diamond pattern of 2*N rows
- 6. Number Diamond Given an integer N as input, write a program to print a number diamond of 2*N -1 r
- 7. Product of the Given NumbersGiven an integer N, write a program which reads N inputs and prints the
- Programming
- Engineering
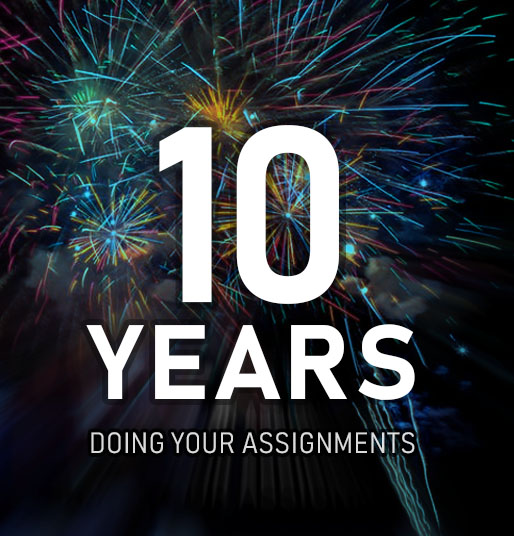
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
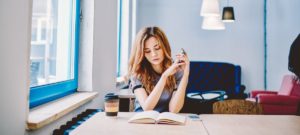
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
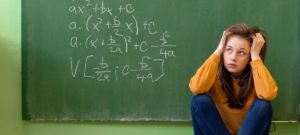
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
5 Best Ways to Create Acronyms in Python
💡 Problem Formulation: When working with text data, it’s common to need to extract an acronym from a phrase or a name. For instance, given the input ‘Asynchronous Javascript and XML’, we desire the output ‘AJAX’. The following article provides various approaches to automate acronym generation in Python.
Method 1: Using List Comprehension and Join
This method involves breaking the string into words, then using list comprehension to extract the first letter of each word and joining these letters together. The split() function is used to break the string into words, and the join() function combines the first letters into the acronym.
Here’s an example:
This function takes a phrase, converts it to uppercase, splits it into words, and then uses list comprehension to generate the acronym by joining the first letter of each word.
Summary/Discussion
- Method 1: List Comprehension and Join. Strengths: concise and straightforward. Weaknesses: less readable for beginners.
- Method 2: Function with For Loop. Strengths: clear and readable, easy to maintain. Weaknesses: more verbose compared to one-liners.
- Method 3: Regular Expressions. Strengths: powerful and capable of handling complex patterns. Weaknesses: can be harder to understand and debug.
- Method 4: Map and Lambda Functions. Strengths: leveraging functional programming, concise. Weaknesses: lambda might be less clear for those unfamiliar with the concept.
- Bonus Method 5: List Comprehension in Function. Strengths: very concise, efficient, and still clear. Weaknesses: may sacrifice some readability for conciseness.
The lambda function takes each word from the split phrase, extracts the first character, converts it to uppercase, and then using the join() function, combines these characters into an acronym.
Bonus One-Liner Method 5: Using List Comprehension in a Function
A compact one-liner inside a function encapsulates the list comprehension technique. This approach is both readable and concise.
The regular expression r'\b\w' finds all the word boundaries followed by an alphanumeric character, which effectively gives us the first letter of each word. These letters are then joined and converted to uppercase to form the acronym.
Method 4: Using map and lambda Functions
The map() function applies a lambda function to each word in the string to extract its first character. This method is useful for one-liners and can also be considered a functional programming approach to the problem.
The generate_acronym function capitalizes the entire phrase, splits it into separate words, then iterates over each word, accumulating the first letter of each to create the acronym, which is finally returned.
Method 3: Using Regular Expressions
Regular expressions provide a powerful way to extract the first letter of each word without explicitly splitting the string. We can use the regex pattern to match the beginning of each word and compile an acronym from these matches.
This code snippet splits the string phrase into a list of words, converts each word to upper case, takes the first character of each, and then joins those characters back into a string to form the acronym.
Method 2: Using a Function with a For Loop
Creating a reusable function that goes through each word in a phrase and compiles an acronym using a for loop gives more control over the process and allows for easy reuse. We define a function generate_acronym() to encapsulate this process.

IMAGES
VIDEO
COMMENTS
Acronyms: You are given some abbreviations as input. Write a program to print the acronyms separated by a dot(.) of those abbreviations. Input: The first line of input contains space-separated strings. Explanation: Consider the given abbreviation, Indian Administrative Service.
Write a Python program to Acronyms, it has two test cases. The below link contains Acronyms question, explanation and test cases. https://docs.google.com/document/d/1tb9wBreT7FpfJwKOEYgFoR1RX-WY7KuD/edit?usp=sharing&ouid=104486799211107564921&rtpof=true&sd=true. We need exact output when the code was run.
Here's how to do acronym with regular expression, leaving numbers as is: IE10. There is a python-based tool for this task, that suggests multiple acronyms, that can be installed via. Source code repo is here: https://github.com/bacook17/acronym. this is my suggestion. print(list1[x][:1],end="")
In this comprehensive Python tutorial, we will demonstrate how to create an acronym generator using Python. While spelling out the whole word takes longer, saying or writing the first initial of each word or an abbreviated form of the full word takes less time.
In this article, we will code a python program to create a dictionary with the key as the first character and value as words starting with that character. Dictionary in Python is an unordered collection of data values, used to store data values like a map, which unlike other Data Types that hold only a single value as an element, Dictionary holds t
Suppose we have a string s that is representing a phrase, we have to find its acronym. The acronyms should be capitalized and should not include the word "and". So, if the input is like "Indian Space Research Organisation", then the output will be ISRO. To solve this, we will follow these steps −.
An acronym is a short form of a word created by long words or phrases such as NLP for natural language processing. In this article, I will walk you through how to write a program to create acronyms using Python.
Python Assignment Operators. Assignment operators are used to assign values to variables:
We need to consider the first character in each of the words to get the acronym. We get the letter I from the first string Indian, we get the letter A from the second word Administrative, and we get the letter S from the third word Service. So, the final output should be I.A.S. abbreviation += word [0] + '.'
The following article provides various approaches to automate acronym generation in Python. This method involves breaking the string into words, then using list comprehension to extract the first letter of each word and joining these letters together.