- C Programming
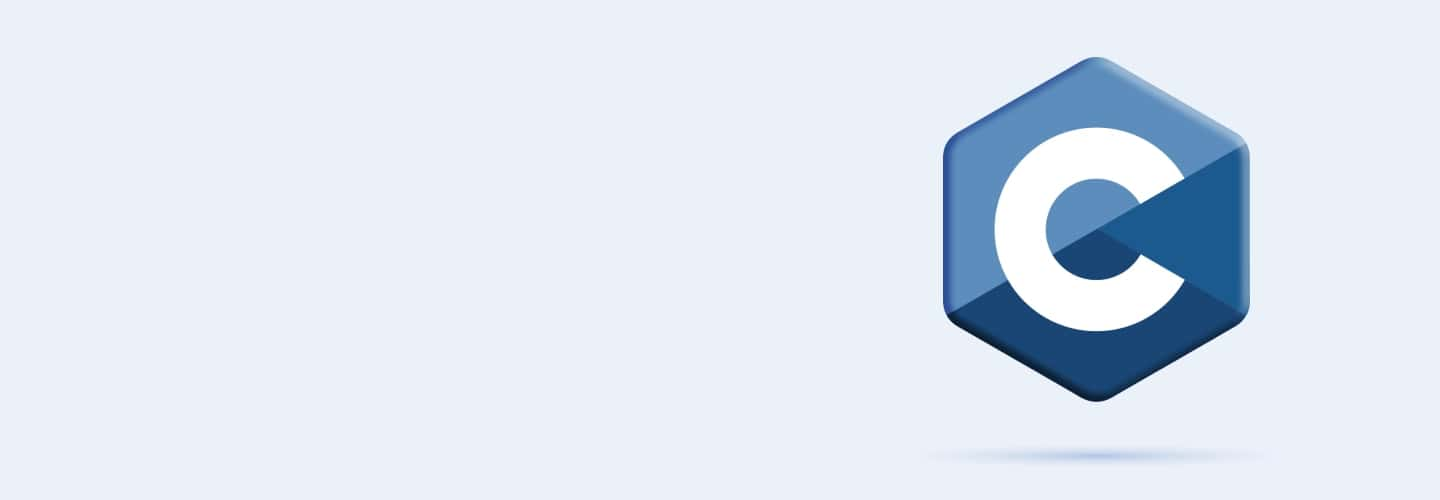

C Programming Multiple Choice Questions (MCQs) and Answers
Master C Programming with Practice MCQs. Explore our curated collection of Multiple Choice Questions. Ideal for placement and interview preparation, our questions range from basic to advanced, ensuring comprehensive coverage of C Programming. Begin your placement preparation journey now!
Q1 Why is learning programming important in today's world?
For academic purposes only
To automate and solve complex problems
Only for software development
For entertainment purposes only
Report a Problem!
Q2 What is the main function of a compiler in programming?
To write code
To interpret code
To convert code into machine language
To debug code
Q3 Which of the following is true about interpreters?
They execute programs faster than compilers
They translate the entire program at once
They translate and execute code line by line
They are not used in modern programming
Q4 How does a compiler differ from an interpreter?
A compiler executes code, while an interpreter does not
A compiler debugs code, while an interpreter does not
A compiler translates the entire program at once, while an interpreter translates it line by line
A compiler is used only in C programming, while an interpreter is not
Q5 What is the primary purpose of writing a "Hello World" program when learning a new programming language?
To test if the language supports string operations
To learn complex programming concepts
To demonstrate basic syntax and output
To introduce advanced programming features
Q6 What will be the output of the following C program? #include <stdio.h> int main() { printf("Hello, World!"); return 0; }
Hello, World!
Syntax Error
Q7 Identify the error in this C program: #include <stdio.h> int main() { print("Hello, World!"); return 0; }
Missing #include statement
Incorrect function name
Syntax error in return statement
Q8 Which of the following is not a keyword in C?
Q9 What data type would you use to store a character in C?
Q10 Which of the following is a valid identifier in C?
none of these
Q11 What is the size of 'int' data type in C?
Depends on the system
Q12 What is the difference between 'float' and 'double' data types in C?
Syntax only
No difference
Q13 What will be the output of the following C code? int main() { char c = 'A'; printf("%d", c); return 0; }
None of these
Q14 Identify the error in this C code: int main() { int number = 100.5; printf("%d", number); return 0; }
Assigning float to int
Syntax error
Wrong printf format
Q15 Spot the error in this code snippet: #include <stdio.h> int main() { const int age; age = 30; printf("%d", age); return 0; }
Uninitialized constant
Missing return statement
Syntax error in printf statement
Q16 What is the purpose of the printf function in C?
To read input
To print output
To perform calculations
To control program flow
Q17 What does %d signify in the printf or scanf function?
Double data type
Decimal integer
Dynamic allocation
Directory path
Q18 What will be the output of the following C code? int main() { printf("%d", 500); return 0; }
Q19 What will the following C code output? int main() { int num; scanf("%d", &num); printf("%d", num); return 0; }
The inputted number
Q20 Pseudocode: READ number PRINT "The number is ", number What does this pseudocode do?
Reads and prints a number
Prints a fixed number
Generates a random number
Q21 Identify the error in this C code: int main() { int x; scanf("%d", x); printf("%d", x); return 0; }
Missing & in scanf
Wrong format specifier
Q22 Find the mistake in this code: int main() { float num; printf("Enter a number: "); scanf("%f", num); printf("You entered: %f", num); return 0; }
Incorrect format specifier in printf
Q23 Which operator is used for division in C?
Q24 What does the '==' operator check?
Greater than
Q25 What is the result of the logical expression (1 && 0)?
Q26 What does the '+' operator do in C?
Subtraction
Multiplication
Q27 Which operator has higher precedence, '+' or '*'?
Both are same
Q28 What does the '!' operator do in C?
Q29 What is the output of the expression 2<3?
Q30 What will be the output of the following C code? int main() { int a = 10, b = 5; printf("%d", a / b); return 0; }
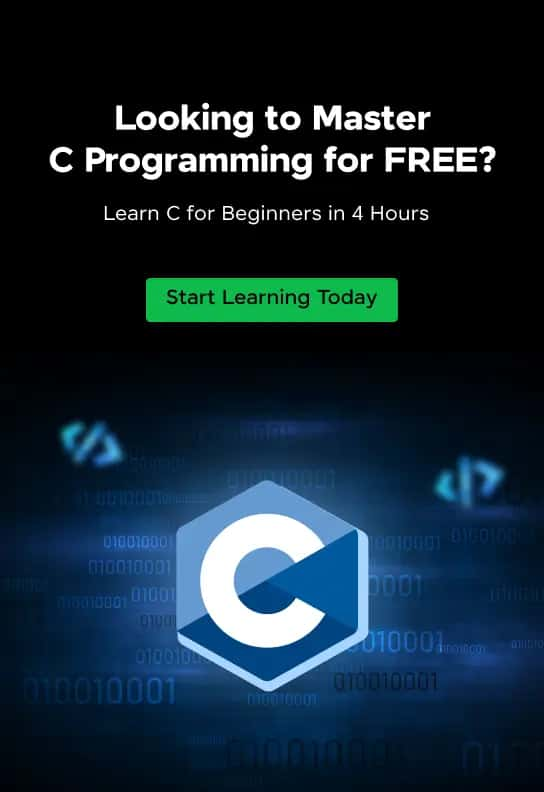
- C Data Types
- C Operators
- C Input and Output
- C Control Flow
- C Functions
- C Preprocessors
- C File Handling
- C Cheatsheet
- C Interview Questions
C Exercises - Practice Questions with Solutions for C Programming
The best way to learn C programming language is by hands-on practice. This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
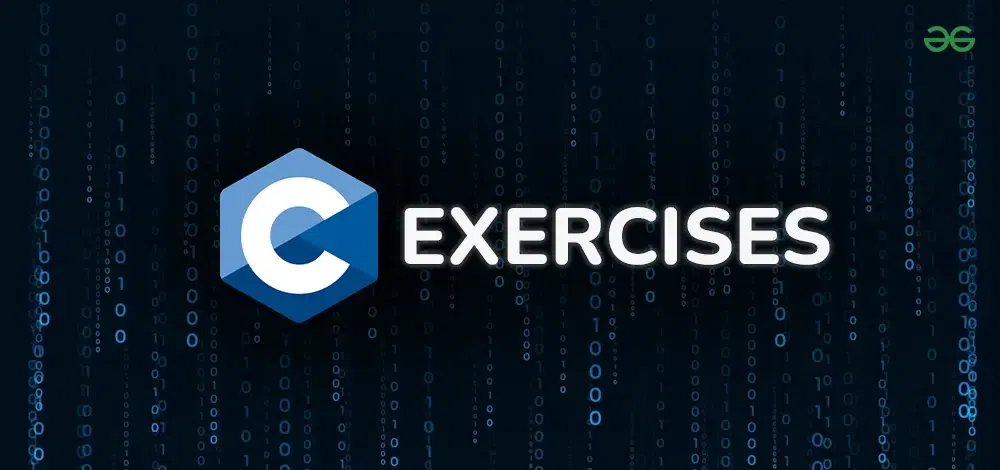
So, Keep it Up! Solve topic-wise C exercise questions to strengthen your weak topics.
Also, Once you've covered basic C exercises, the GeeksforGeeks Practice Platform is a great place to take on more advanced C coding problems and improve your understanding.
C Programming Exercises
The following are the top 30 programming exercises with solutions to help you practice online and improve your coding efficiency in the C language. You can solve these questions online in GeeksforGeeks IDE.
Q1: Write a Program to Print "Hello World!" on the Console.
In this problem, you have to write a simple program that prints "Hello World!" on the console screen.
For Example,
Click here to view the solution.
Q2: write a program to find the sum of two numbers entered by the user..
In this problem, you have to write a program that adds two numbers and prints their sum on the console screen.
Q3: Write a Program to find the size of int, float, double, and char.
In this problem, you have to write a program to print the size of the variable.
Q4: Write a Program to Swap the values of two variables.
In this problem, you have to write a program that swaps the values of two variables that are entered by the user.
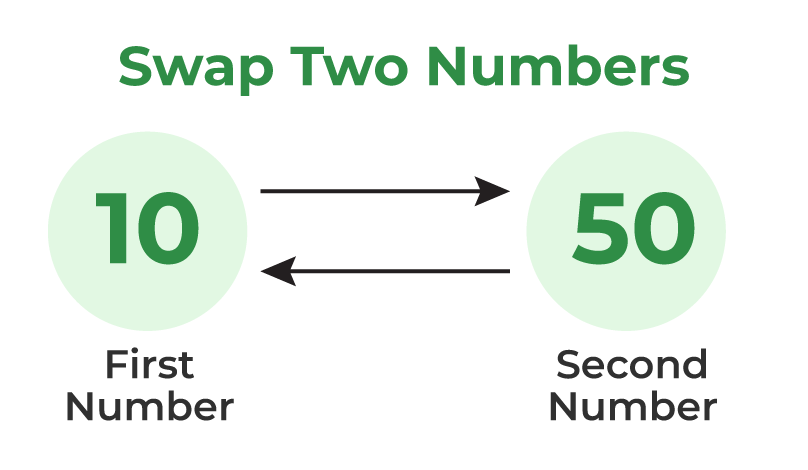
Q5: Write a Program to calculate Compound Interest.
In this problem, you have to write a program that takes principal, time, and rate as user input and calculates the compound interest.
Q6: Write a Program to check if the given number is Even or Odd.
In this problem, you have to write a program to check whether the given number is even or odd.
Q7: Write a Program to find the largest number among three numbers.
In this problem, you have to write a program to take three numbers from the user as input and print the largest number among them.
Q8: Write a Program to make a simple calculator.
In this problem, you have to write a program to make a simple calculator that accepts two operands and an operator to perform the calculation and prints the result.
Q9: Write a Program to find the factorial of a given number.
In this problem, you have to write a program to calculate the factorial (product of all the natural numbers less than or equal to the given number n) of a number entered by the user.
Q10: Write a Program to Convert Binary to Decimal.
In this problem, you have to write a program to convert the given binary number entered by the user into an equivalent decimal number.
Q11: Write a Program to print the Fibonacci series using recursion.
In this problem, you have to write a program to print the Fibonacci series(the sequence where each number is the sum of the previous two numbers of the sequence) till the number entered by the user using recursion.
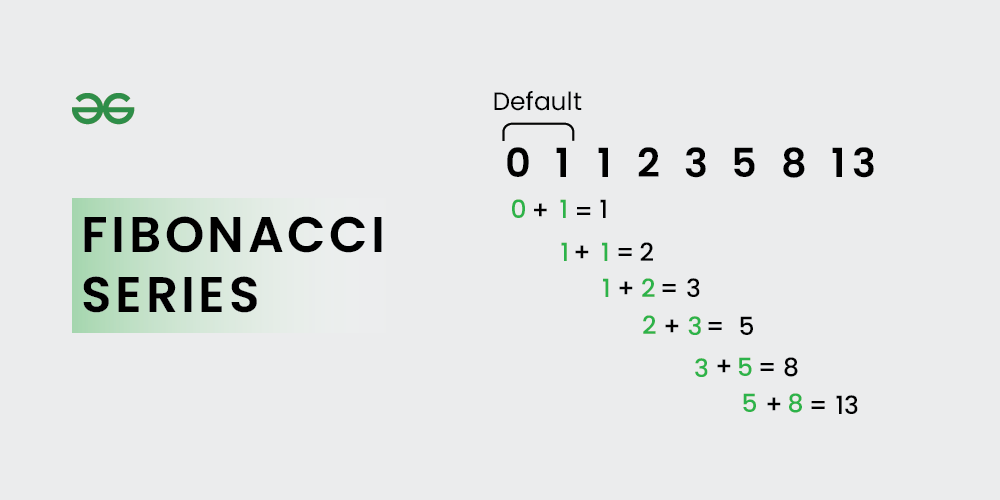
Q12: Write a Program to Calculate the Sum of Natural Numbers using recursion.
In this problem, you have to write a program to calculate the sum of natural numbers up to a given number n.
Q13: Write a Program to find the maximum and minimum of an Array.
In this problem, you have to write a program to find the maximum and the minimum element of the array of size N given by the user.
Q14: Write a Program to Reverse an Array.
In this problem, you have to write a program to reverse an array of size n entered by the user. Reversing an array means changing the order of elements so that the first element becomes the last element and the second element becomes the second last element and so on.
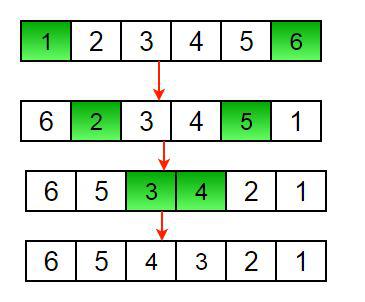
Q15: Write a Program to rotate the array to the left.
In this problem, you have to write a program that takes an array arr[] of size N from the user and rotates the array to the left (counter-clockwise direction) by D steps, where D is a positive integer.
Q16: Write a Program to remove duplicates from the Sorted array.
In this problem, you have to write a program that takes a sorted array arr[] of size N from the user and removes the duplicate elements from the array.
Q17: Write a Program to search elements in an array (using Binary Search).
In this problem, you have to write a program that takes an array arr[] of size N and a target value to be searched by the user. Search the target value using binary search if the target value is found print its index else print 'element is not present in array '.
Q18: Write a Program to reverse a linked list.
In this problem, you have to write a program that takes a pointer to the head node of a linked list, you have to reverse the linked list and print the reversed linked list.
Q18: Write a Program to create a dynamic array in C.
In this problem, you have to write a program to create an array of size n dynamically then take n elements of an array one by one by the user. Print the array elements.
Q19: Write a Program to find the Transpose of a Matrix.
In this problem, you have to write a program to find the transpose of a matrix for a given matrix A with dimensions m x n and print the transposed matrix. The transpose of a matrix is formed by interchanging its rows with columns.
Q20: Write a Program to concatenate two strings.
In this problem, you have to write a program to read two strings str1 and str2 entered by the user and concatenate these two strings. Print the concatenated string.
Q21: Write a Program to check if the given string is a palindrome string or not.
In this problem, you have to write a program to read a string str entered by the user and check whether the string is palindrome or not. If the str is palindrome print 'str is a palindrome' else print 'str is not a palindrome'. A string is said to be palindrome if the reverse of the string is the same as the string.
Q22: Write a program to print the first letter of each word.
In this problem, you have to write a simple program to read a string str entered by the user and print the first letter of each word in a string.
Q23: Write a program to reverse a string using recursion
In this problem, you have to write a program to read a string str entered by the user, and reverse that string means changing the order of characters in the string so that the last character becomes the first character of the string using recursion.
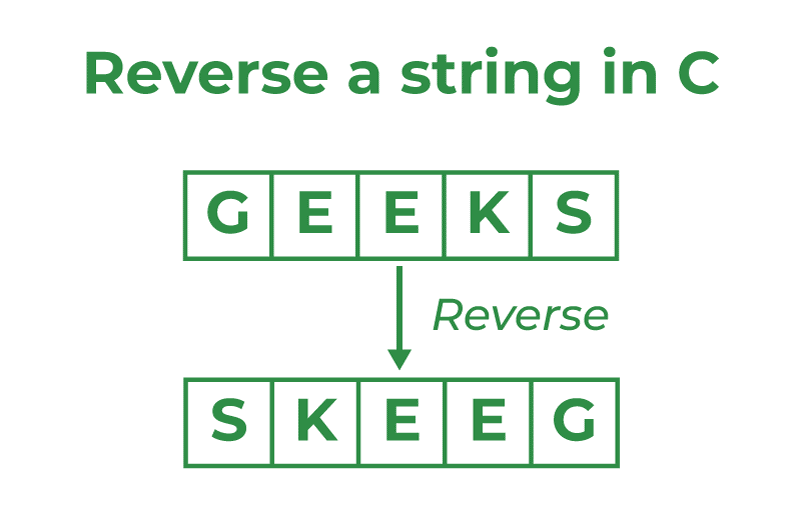
Q24: Write a program to Print Half half-pyramid pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print the half-pyramid pattern of numbers. Half pyramid pattern looks like a right-angle triangle of numbers having a hypotenuse on the right side.
Q25: Write a program to print Pascal’s triangle pattern.
In this problem, you have to write a simple program to read the number of rows (n) entered by the user and print Pascal’s triangle pattern. Pascal’s Triangle is a pattern in which the first row has a single number 1 all rows begin and end with the number 1. The numbers in between are obtained by adding the two numbers directly above them in the previous row.
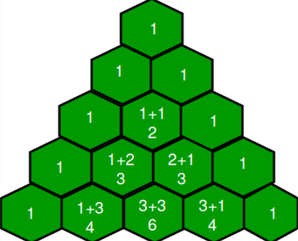
Q26: Write a program to sort an array using Insertion Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending or descending order using insertion sort.
Q27: Write a program to sort an array using Quick Sort.
In this problem, you have to write a program that takes an array arr[] of size N from the user and sorts the array elements in ascending order using quick sort.
Q28: Write a program to sort an array of strings.
In this problem, you have to write a program that reads an array of strings in which all characters are of the same case entered by the user and sort them alphabetically.
Q29: Write a program to copy the contents of one file to another file.
In this problem, you have to write a program that takes user input to enter the filenames for reading and writing. Read the contents of one file and copy the content to another file. If the file specified for reading does not exist or cannot be opened, display an error message "Cannot open file: file_name" and terminate the program else print "Content copied to file_name"
Q30: Write a program to store information on students using structure.
In this problem, you have to write a program that stores information about students using structure. The program should create various structures, each representing a student's record. Initialize the records with sample data having data members' Names, Roll Numbers, Ages, and Total Marks. Print the information for each student.
We hope after completing these C exercises you have gained a better understanding of C concepts. Learning C language is made easier with this exercise sheet as it helps you practice all major C concepts. Solving these C exercise questions will take you a step closer to becoming a C programmer.
Frequently Asked Questions (FAQs)
Q1. what are some common mistakes to avoid while doing c programming exercises.
Some of the most common mistakes made by beginners doing C programming exercises can include missing semicolons, bad logic loops, uninitialized pointers, and forgotten memory frees etc.
Q2. What are the best practices for beginners starting with C programming exercises?
Best practices for beginners starting with C programming exercises: Start with easy codes Practice consistently Be creative Think before you code Learn from mistakes Repeat!
Q3. How do I debug common errors in C programming exercises?
You can use the following methods to debug a code in C programming exercises Read the error message carefully Read code line by line Try isolating the error code Look for Missing elements, loops, pointers, etc Check error online
Similar Reads
Improve your coding skills with practice.
What kind of Experience do you want to share?

100+ C Language Solved MCQs
These multiple-choice questions (MCQs) are designed to enhance your knowledge and understanding in the following areas: Bachelor of Business Administration in Computer Applications (BBA [CA]) .
- Introduction to C Language
- Managing IO Operations
Done Studing? Take A Test.
Great job completing your study session! Now it's time to put your knowledge to the test. Challenge yourself, see how much you've learned, and identify areas for improvement. Don’t worry, this is all part of the journey to mastery. Ready for the next step? Take a quiz to solidify what you've just studied.

- Data Structure
- Coding Problems
- C Interview Programs
- C++ Aptitude
- Java Aptitude
- C# Aptitude
- PHP Aptitude
- Linux Aptitude
- DBMS Aptitude
- Networking Aptitude
- AI Aptitude
- MIS Executive
- Web Technologie MCQs
- CS Subjects MCQs
Databases MCQs
Programming MCQs
Testing Software MCQs
- Digital Mktg Subjects MCQs
- Cloud Computing S/W MCQs
Engineering Subjects MCQs
- Commerce MCQs
- More MCQs...
- Machine Learning/AI
- Operating System
- Computer Network
- Software Engineering
- Discrete Mathematics
- Digital Electronics
- Data Mining
- Embedded Systems
- Cryptography
- CS Fundamental
- More Tutorials...
- Tech Articles
- Code Examples
- Programmer's Calculator
- XML Sitemap Generator
- Tools & Generators
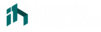
Multiple-Choice Questions
- MCQs - HOme
Web Technologies MCQs
- JavaScript MCQs
- jQuery MCQs
- ReactJS MCQs
- AngularJS MCQs
- Advanced CSS MCQs
- CHERRYPY MCQs
- ZEND FRAMEWORK MCQs
- KNOCKOUTJS MCQs
- EMBERJS MCQs
- NEXT.JS MCQs
- LARAVEL MCQs
- BACKBONEJS MCQs
- APACHE TAPESTRY MCQs
- FUELPHP MCQs
- EXPRESS.JS MCQs
- JASMINE.JS MCQs
- KOA.JS MCQs
- AURELIA MCQs
- BABYLON.JS MCQs
- METEOR.JS MCQs
- YII FRAMEWORK MCQs
- ELECTRON.JS MCQs
- EXT.JS MCQs
- SYMFONY MCQs
- MOOTOOLS MCQs
- LEAFLET MCQs
- PHALCON MCQs
- LODASH MCQs
- CODEIGNITER MCQs
- FOUNDATION MCQs
- FASTAPI MCQs
- RUBY ON RAILS MCQs
- MEAN STACK MCQs
- CPANEL MCQs
- FULL STACK DEVELOPMENT MCQs
Computer Science Subjects MCQs
- S/W ENGINEERING MCQs
- DATA ANALYTICS MCQs
- CRYPTOGRAPHY MCQs
- BLOCKCHAIN MCQs
- COMPUTER GRAPHICS MCQs
- EMBEDDED SYSTEM MCQs
- BIG DATA ANALYTICS MCQs
- DATA ANALYTICS & VISUALIZATION MCQs
- OPERATING SYSTEM MCQs
- COMPUTER AWARENESS
- PROJECT MANAGEMENT MCQs
- COMPUTER NETWORK MCQs
- THEORY OF COMPUTATION MCQs
- COMPUTER ORG.& ARCH. MCQs
- DIGITAL CIRCUITS MCQs
- MICROPROCESSOR MCQs
- CYBER SECURITY MCQs
- Algorithms MCQs
- BLOGGING MCQs
- WORDPRESS MCQs
- INTERNET & EMAIL MCQs
- COMPUTER MEMORY MCQs
- TYPES OF COMPUTERS MCQs
- PL/SQL MCQs
- ORACLE MCQs
- MONGODB MCQs
- SQLITE MCQs
- COUCHDB MCQs
- MARIADB MCQs
- ARANGODB MCQs
- APACHE PRESTO MCQs
- APACHE DERBY MCQs
- Transact-SQL (T-SQL) MCQs
- POUCHDB MCQs
- HSQLDB MCQs
- ORIENTDB MCQs
- DYNAMODB MCQs
- TINYDB MCQs
- MEMCACHED MCQs
- HAZELCAST MCQs
- IMS DB MCQs
- INDEXEDDB MCQs
- C LANGUAGE MCQs
- C++ LANGUAGE MCQs
- PYTHON MCQs
- ASP.NET MCQs
- JAVA SWING MCQs
- SPRING MCQs
- APACHE ANT MCQs
- APACHE IVY MCQs
- APACHE ACTIVEMQ MCQs
- APACHE NIFI MCQs
- APACHE CAMEL MCQs
- JACKSON MCQs
- EASYMOCK MCQs
- ETL TESTING MCQs
- BUGZILLA MCQs
- SOFTWARE TESTING MCQs
- SELENIUM MCQs
- AGILE METHODOLOGY MCQs
- JMETER MCQs
- APPIUM MCQs
- CUCUMBER TESTING MCQs
- UIPATH MCQs
- TESTNG MCQs
Digital Marketing Subjects MCQs
- DIGITAL MARKETING MCQs
- ONLINE MARKETING MCQs
- AFFILIATE MARKETING MCQs
- GOOGLE ADWORDS MCQs
- GOOGLE ADS PPC MCQs
- FACEBOOK ADS MCQs
- INSTAGRAM MARKETING MCQs
Cloud Computing Softwares MCQs
- OPENSTACK MCQs
- Microsoft Azure MCQs
- Google Cloud Platform MCQs
- DOCKER MCQs
- OPENSHIFT MCQs
AI/ML Subjects MCQs
- ARTIFICIAL INTELLIGENCE
- REINFORCEMENT LEARNING
- PYSPARK MCQs
- PYBRAIN MCQs
- DATA SCIENCE MCQs
- STATISTICS MCQs
- DATA & INFORMATION
- AutoCAD MCQs
- Discrete Mathematics MCQs
- Environment Engineering MCQs
- CONTROL SYSTEMS MCQs
- Software Architecture MCQs
- Microwave Engineering MCQs
- Network Theory MCQs
- Electrical Machines MCQs
- Renewable Energy MCQs
- Satellite Communication MCQs
- Mechatronics MCQs
- Corrosion Eng. MCQs
- Wireless & Mobile Comm. MCQs
- Audio Video Engineering MCQs
- Antenna MCQs
- Steam & Gas Turbines MCQs
- Basic Electrical Engineering MCQs
- Material Science MCQs
- Total Quality Management MCQs
- Engineering Geology MCQs
- Engineering Metrology MCQs
- Fluid Mechanics MCQs
- Engineering Mechanics MCQs
- Strength of Materials MCQs
- Structural Analysis MCQs
- Design of Steel Structures MCQs
- Estimating & Costing MCQs
- Hydrology MCQs
- Building Construction & Materials MCQs
- Irrigation Engineering MCQs
- Highway Engineering MCQs
Office Related Programs MCQs
- MICROSOFT WORD MCQs
- MICROSOFT EXCEL MCQs
- MICROSOFT POWERPOINT MCQs
- GOOGLE SHEETS MCQs
Management MCQs
- Marketing MCQs
- Marketing 4.0 MCQs
- Management Information System (MIS) MCQs
- Consumer Behaviour MCQs
- Supply Chain Management (SCM) MCQs
- DATA PRIVACY MCQs
- GOOGLE CHROME MCQs
- SQLAlchemy MCQs
- APACHE FLINK MCQs
- SWAGGER MCQs
- SOAPUI MCQs
Home » MCQs
C Programming Language MCQs
C is a general-purpose, procedural computer programming language, C language supports structured programming, lexical variable scope, and recursion, with a static type system. C language is used to develop software like operating systems, databases, compilers, and so on.
C language MCQs : This section contains multiple-choice questions and answers on the C programming language . It will help the students to test their skills and prepare well for their exams.
List of C programming MCQs
- C Basics MCQs
- C Data Types, Operators and Expressions MCQs
C Conditional Statements MCQs
- C Control Statements MCQs
- C Strings MCQs
- C Arrays MCQs
- C Structures and Union MCQs
- C Functions MCQs
- C Pointers MCQs
- C File Handling MCQs
- C Preprocessor MCQs
1) C Basics MCQs
1. c language was developed by ___..
- Dennis Rechard
- Dennis M. Ritchie
- Bjarne Stroustrup
- Anders Hejlsberg
B) Dennis M. Ritchie
Explanation
C programming language was developed by an American computer scientist Dennis M. Ritchie at Bell Laboratories (formerly AT&T Bell Laboratories).
2. In which year was C language developed?
C programming language was developed by Dennis Ritchie at Bell Laboratories in 1972.
3. C language is a successor to which language?
C programming language is a successor to the programming language B.
4. C is a ___.
- Low level language
- High level language
- Medium level language
- None of the above
C) Medium level language
C is a medium-level language because it contains the features of low-level language as well as high-level language.
5. How many keywords are there in C language?
C language has only 32 keywords.
6. C language is a ___.
- Procedural oriented programming language
- General purpose programming language
- Structured programming
- All of the above
D) All of the above
C programming language is a general-purpose, procedural computer programming language that supports structured programming also.
7. Which is not a valid keyword in C language?
C) do-while
do-while is not a valid keyword in the C programming language. It's a control statement. 'do' and 'while' are the separate keywords. The rest of all 'for', 'while', and 'switch' are the valid keywords in C.
8. What is an identifier in C language?
- An identifier is a combination of alphanumeric characters used for conditional and control statements
- An identifier is a combination of alphanumeric characters used for any variable, function, label name
- Both A and B
B) An identifier is a combination of alphanumeric characters used for any variable, function, label name
An identifier is a combination of alphanumeric characters used for any variable, function, label name. An identifier is a name that is used to identify the variables/ constants, functions, arrays, label name, and user-defined data.
9. A C-style comment, simply surround the text with ___.
- /** and **/
A) /* and */
A C-style comment , simply surround the text with /* and */.
10. Can we place comments between the statement to comments a part of the code?
Yes, we can place comments between the statement to comments a part of the code.
11. ___ is an informal name for ISO/IEC 9899:1999, a past version of the C programming language standard?
C99 is an informal name for ISO/IEC 9899:1999, a past version of the C programming language standard.
12. In which version of C language, the C++ Style comment (//) are introduced?
C language version C99 introduced C++ style comment (//), they can be used to comment a single line.
13. The C source file is processed by the ___.
- Interpreter
- Both Interpreter and Compiler
B) Compiler
The C source file is processed by the compiler.
14. How many whitespace characters are allowed in C language?
There are 5 whitespace characters are allowed in C language, they are:
- Horizontal tab
- Vertical tab
15. How many punctuation characters are allowed in C language?
There are 29 punctuation characters are allowed in C language, they are:
16. What is the extension of a C language source file?
The extension of a C language source file is ".c".
17. What is the extension of a C language header file?
The extension of a C language source file is ".h".
18. To develop which operating, C language was invented?
C language was invented to develop Unix operating system.
19. Does C language support object-oriented approach?
C language does not support object-oriented approach.
20. Which is/are the disadvantage(s) of C language?
- No Garbage Collection
- Inefficient Memory Management
- Low level of abstraction
- Lack of Object Orientation
E) All of the above
The main disadvantages of C language are:
2) C Data Types, Operators and Expressions MCQs
21. which are the fundamental data types in c.
The fundamental / basic data types in C language :
22. How many byte(s) does a char type take in C?
The char data type takes one byte in the memory.
23. For which type, the format specifier "%i" is used?
In C programming language, both of the format specifier %d and %i are used for int type, where %d specifies the type of variable as decimal and %i specifies the type as integer.
24. What is the difference between float and double in C?
- both are used for the same purpose
- double can store just double value as compare to float value
- double is an enhanced version of float and was introduced in C99
- double is more precise than float and can store 64 bits
D) double is more precise than float and can store 64 bits
In C programming language, the double is more precise than float and can store 64 bits.
25. Which is the correct format specifier for double type value in C?
The %lf is used to represent a double type value in C programming language.
26. The short type represents ___.
- unsigned int
C) unsigned int
The short type represents short int in C language.
27. How many byte(s) does a short type take in C?
In C programming language, the short or short int takes 2 bytes (16 bits) in memory.
28. What is the correct syntax to declare a variable in C?
- data_type variable_name;
- data_type as variable_name;
- variable_name data_type;
- variable_name as data_type;
A) data_type variable_name;
In C language, the correct syntax to declare a variable is:
Where, data_type is the type of data (such as int , char , float , etc) and variable_name is a valid identifier . For example: int age;
29. How many types of qualifiers are there in C language?
There are 3 types of qualifiers in C language, they are:
- Size qualifiers
- Sign qualifiers
- Type qualifiers
30. Which is/are the size qualifier(s) in C language?
- Both A. and B
D) Both A. and B.
The size qualifiers are short and long .
31. Which is/are the sign qualifier(s) in C language?
The sign qualifiers are used to specify the signed nature of an integer type. The sign qualifiers are signed and unsigned .
32. Which is/are the type qualifier(s) in C language?
The type qualifiers are const and "volatile".
33. Which is correct with respect to the size of the data types in C?
- char > int > float
- char < int < float
- int < char < float
- int < chat > float
B) char < int < float
The correct order of the data types as per the size is: char < int > float .
34. Which operator is used to find the remainder of two numbers in C?
The % operator is known as "Modulus Operator" and it is used to find the remainder of two numbers.
35. Which of the following is not an arithmetic expression?
x != 10 is not a valid arithmetic expression.
36. What will be the output of the following C code?
In the above code, the value of x is 20 and then in the next statement, the expression is x %= 3 . That will be evaluate as:
37. What will be the output of the following C code?
In the above code, we are performing modulus operation with float values. Modulus operator doesn't work with float and double operands. Thus, the output will be:
38. What will be the output of the following C code?
In the above code, the value of x is 23.456 and we are printing the value of x using the %.2f format specifier. %.2f rounds the value and prints the 2 digits after the decimal point.
39. What will be the output of the following C code?
In the above code, we are using a post-increment statement ( x++ ), post-increment increases the value after evaluating the current expression. Thus, the value of y will be 30 and then x will be 11.
40. Increment (++) and decrement (--) are the ___ operators in C?
Increment (++) and decrement (--) are the unary operators. They need one operand to perform the operation.
41. What will be the output of the following C code?
290 is beyond the range of unsigned char . Its corresponding value printed is: (290 % (UCHAR_MAX +1) where UCHAR_MAX represents highest (maximum) value of unsigned char type of variable. The value of UCHAR_MAX=255. Thus it prints 290 % (UCHAR_MAX+1)=34
42. What will be the output of the following C code?
Bitwise OR operator (|) has precedence over logical OR operator (||). Thus the expression 5 || 2 | 1 is actually 5 || (2 |1).
43. What will be the output of the following C code?
The statement "-100;" is evaluated and this does not affect the value of "x".
44. What will be the output of the following C code?
0x10 is hex value it's decimal value is 16 and 010 is an octal value it's decimal value is 8, hence answer will be 24.
45. Which C keyword is used to extend the visibility of variables?
The "extern: keyword used to define an extern variable , that can be accessed in any source file. i.e., extern is used to extend the visibility of variables in C language.
46. What is the name of "&" operator in C?
C) Address of
The " & " operator is known as 'Address Of' operator which is used to access the address of a variable.
47. Which of the following are valid decision-making statements in C?
- All of these
D) All of these
All valid decision-making statements in C program are:
- if statement
- if-else statement
- nested if statement
- switch statement
- nested switch statement
48. Decision making in the C programming language is ___.
- Repeating the same statement multiple times
- Executing a set of statements based on some condition
- Providing a name of the block of code
B) Executing a set of statements based on some condition
Decision-making in C programming is executing a block of code to be executed by the program based on some condition.
49. Which of the following is a true value in C programming?
- "includehelp"
All non-zero and non-null values in C programming are true.
50. Ternary operator in C programming is ___.
- None of these
Ternary operator is used to execute expressions based on the given condition.
51. What will be the output of the following C code?
- value 1 is not greater
- value 1 is greater
A) value 1 is not greater
52. What is the correct syntax of if statement in C program?
- if(condition){ }
- if(condition) :
- If { [condition] }
The correct syntax of if statement in C program is:
53. The if statement is a conditional statement?
The if statement is a conditional statement, i.e., the block is executed based on the given condition.
54. When the condition of if statement is false, the flow of code will ___.
- go into the if block
- Exit the program
- Continue the code after skipping the if block
C) Continue the code after skipping the if block
When the condition of if statement is false, the code after the if block will be executed and the if block code is skipped.
55. What will be the result of the following condition?
56. which statement is required to execute a block of code when the condition is false.
In the if-else block, the if block is executed when condition is True and else block is executed when condition is false.
57. Can the else statement exist without the if statement in C?
The else statement needs an if statement to execute the block.
58. Which of these if...else block syntax is correct?
- if(condition){ } else { }
- if(condition){ } else(condition){ }
- if{ } else { }
The syntax of if...else block of code in C is:
59. The if-elseif-else statement in C programming is used?
- Create multiple conditional statements
- Return values
- Loop in if-else block
A) Create multiple conditional statements
The if-elseif-else statement is a statement which contains multiple statements based on conditions.
60. What will be the output of the following C code?
D) Grade : D
61. How many expressions can be checked using if...elseif...else statement?
C) Infinite
You can execute any number of expressions using if-elseif-else statements, each elseif statement containing one condition.
62. Is it possible to nest if-else statements in C programming?
Nesting of if-else statements in C programming is possible.

63. Which of the following syntax is correct for nested if-else statements?
- if(exp1){ if(exp2){ } } else { if(exp3){ } }
- if(exp1){ }else { }
The correct syntax for nested if-else statements in C programming is:
64. What will be the output of the following C code?
65. multiple values of the same variable can be tested using ___..
The switch statement in C is used to test for multiple values of a variable.
66. Without a break statement in switch what will happen?
- All cases will work properly
- Cases will fall through after matching the first check
- Switch will throw error
B) Cases will fall through after matching the first check
The break statement is used to terminate the current flow of code. And if it is not present in the switch statement, the cases will execute all cases after the matched case.
67. When all cases are unmatched which case is matched in a switch statement?
- Default case
A) Default case
The default case of switch statement is executed when no other case is matched.
68. What will be the output of the following C code?
- Better try again
There is no break statement in case B, Case C, case D. the code will fall through executing all the print statements.
4) C Control Statements MCQs
69. loops in c programming are used to ___..
- Execute a statement based on a condition
- Execute a block of code repeatedly
- Create a variable
B) Execute a block of code repeatedly
Loops in programming are used to execute a block of code repeatedly.
70. Which of these is an exit-controlled loop?
C) do...while
The do...while loop check for a condition after executing the loop block once. Hence, it is called an exit-controlled loop.
71. Which statements are used to change the execution sequence?
- Loop control statement
- Function statement
- Conditional statement
A) Loop control statement
Loop control statements in C are used to change the execution sequence of the loop.
72. What will happen if the loop condition will never become false?
- Program will throw an error
- Program will loop infinitely
- Loop will not run
B) Program will loop infinitely
An infinite loop in a program is a condition when the loop continues when the loop continues to run infinitely because the condition never becomes false.
73. Which of these statements is correct in case of while loop in C?
- Executes the block till the condition become false
- Is an entry controlled loop
- There might be condition when the loop will not execute at all
All of the above statements are true in the case of while loop. While loop is an entry-controlled loop, the block executes when the condition becomes false.
74. Which of the following is valid syntax for creating a while loop?
- while{ } (condition)
- while(condition){ }
The correct syntax for creating a while loop is:
75. What will be the output of the following C code?
- 11 13 15 17 19
- 11 12 13 14 15 16 17 18 19 20
- 11 13 15 17 19 21
A) 11 13 15 17 19
76. Which loop executes the block a specific number of times?
- do...while loop
B) for loop
The for loop executes the block a specific number of times.
77. Which of the following parts of the for loop can be eliminated in C?
Syntax of for loop:
Inside the initialization statement (init), any of the three init or condition or increment can be eliminated i.e., all are optional. The loop can work without them also.
78. When all parts of the for loop are eliminated, what will happen?
- For loop will not work
- Infinite for loop
B) Infinite for loop
On eliminating all the parts of a for loop an infinite for loop will run.
79. When the condition of the do-while loop is false, how many times will it execute the code?
The do-while loop is an exit-controlled loop, hence it will run at least once, even if the condition becomes false.
80. Can a loop be nested in C programming?
Yes, the nesting of loop is possible in C programming language.
81. What will be the output of the following C code?
- 2 3 4 5 6 7 8 9
5) C Strings MCQs
82. a string is terminated by ___..
- Newline ('\n')
- Null ('\0')
B) Null ('\0')
In C programming language, a string is a sequence of characters terminated with a null character \0.
83. Consider the below statement, can we assign a string to variable like this:
No, we cannot assign a string like this. Because string is a character array and array type is not assignable. To assign a string to the character array, we need to use strcpy() function.
84. Which format specifier is used to read and print the string using printf() and scanf() in C?
The format specifier "%s" is used to read and print the string using printf() and scanf() in C.
85. Which function is used to read a line of text including spaces from the user in C?
In C programming language, the fgets() function can be used to read a line of text including spaces from the user.
86. Which function is used to concatenate two strings in C?
- stringcat()
D) strcat()
In C programming language, the strcat() function is used to concatenate two strings.
This function concatenates string s2 onto the end of string s1.
87. What will be the output of the following C code?
str1 is initialized with the characters and there are only 5 characters. Thus, the length of the str is 5. While, str2 is initialized with the string "Hello", when we initialized the string in this way - a null ('\0') character is inserted after the string. Thus, the length of str2 is 6.
88. What will be the output of the following C code?
- Hello,Hello
- Hello,HelloHello
There will be a compilation error, because we cannot assign a string like this (str2 = str1). To resolve this issue, we have to use strcpy(str2,str1).
The output will be:
89. What will be the output of the following C code? (If the input is "Hello world")
- Hello world
- Hello world\0
When we read a string using the scanf() function, the input is terminated by the whitespace. Here, the input is "Hello world", so only "Hello" will be stored to str . Thus, the output will be "Hello".
90. Which function is used to compare two strings in C?
A) strcmp()
The function strcmp() is used to compare two strings in C language.
The strcmp() is a built-in library function and is declared in <string.h> header file . This function takes two strings as arguments and compare these two strings lexicographically.
91. Which function is used to compare two strings with ignoring case in C?
B) strcmpi()
The function strcmpi() is used to compare two strings with ignoring case in C language.
The strcmpi() is a built-in library function and is declared in <string.h> header file. This function takes two strings as arguments and compare these two strings, it is similar to strcmp() function but the only difference is that strcmpi() function is not case sensitive.
6) C Arrays MCQs
92. which is the correct syntax to declare an array in c.
- data_type array_name[array_size];
- data_type array_name{array_size};
- data_type array_name[];
A) data_type array_name[array_size];
The correct syntax to declare an array in C is:
93. You can access elements of an array by ___.
- memory addresses
You can access elements of an array by indices. Array indices starts from 0 to array_size -1.
94. Which is/are the correct syntax to initialize an array in C?
- data_type array_name[array_size] = {value1, value2, value3, …};
- data_type array_name[] = {value1, value2, value3, …};
- data_type array_name[array_size] = {};
D) Both A and B
Both the options A and B are correct to initialize an array.
95. Array elements are always stored in ___ memory locations.
B) Sequential
Array elements are always stored in sequential memory locations.
96. Let x is an integer array with three elements having value 10, 20, and 30. What will be the output of the following statement?
- Prints the value of 0 th element (i.e., 10)
- Prints the garbage value
- An error occurs
- Print the address of the array (i.e., the address of first (0 th ) element
D) Print the address of the array (i.e., the address of first (0 th ) element
If we print the array (in this case, x). The output will be the memory address of the array (i.e., the address of first (0 th ) element.
97. What will be the output of the following C program?
- Garbage value
In C language, when an array is partially initialized at the time of declaration then the remaining elements of the array is initialized to 0 by default.
98. What will be the output of the following C program?
C) Garbage value
C language compiler does not check array with its bounds, when an index is out of the range the garbage value is printed.
99. What will be the output of the following C program?
The statement sizeof(x)/sizeof(x[0]) can be used to get the total numbers of elements, sizeof(x) will return the size of array while sizeof(x[0]) will return the size of first element i.e., size of the type. Their division will return the total number of elements.
100. What will be the output of the following C program?
101. what will be the output of the following c program, 102. if we pass an array as an argument to a function, what actually gets passed.
- Value of elements in array
- First element of the array
- Base address of the array i.e., the address of the first element
- Address of the last element of array
C) Base address of the array i.e., the address of the first element
If we pass an array as an argument to a function – the base address of the array (the address of the first element) is passed to the function.
7) C Structures and Union MCQs
103. which of the following is the collection of different data types.
A) structure
In C programming language, a structure is a user-defined data type which is the collection of different data types.
104. Which operator is used to access the member of a structure?
The dot (.) operator is used to access the member of a structure.
105. Which of these is a user-defined data type in C?
Union is a user defined data type in C.
106. "A union can contain data of different data types". True or False?
Union is a user defined data structure which can store data variables of different data types.
107. Which keyword is used to define a union?
The union is defined using the keyword union.
108. The size of a union is ___.
- Sum of sizes of all members
- Predefined by the compiler
- Equal to size of largest data type
C) Equal to size of largest data type
The size of the union is equal to the size of the largest data type declared in the union.
109. All members of union ___.
- Stored in consecutive memory location
- Share same memory location
- Store at different location
B) Share same memory location
All members of a union in C are stored at the same memory location.
110. Which of the below statements is incorrect in case of union?
- Union is a user-defined data structure
- All data share same memory
- Union stores methods too
- union keyword is used to initialize
C) Union stores methods too
Unions in C is a data structure which store data types but not the methods.
111. The members of union can be accessed using ___.
- Dot Operator (.)
- And Operator (&)
- Asterisk Operator (*)
- Right Shift Operator (>)
A) Dot Operator (.)
The dot operator (.) is used to access members of a union.
112. In which case union is better than structure?
- Less memory is available
- Faster compilation is required
- When functions are included
A) Less memory is available
113. Which is the correct syntax to create a union?
- union union_name { };
- union union_name { }
- union union_name ( );
- Union union_name ( )
The correct syntax of creating a union is:
114. What will be the output of the following C program?
- val1 = 0x54ac88dd2012 val2 = 0x55ac76dd2014
- val1 = 0x55ac76dd2014 val2 = 0x55ac76dd2014
C) val1 = 0x55ac76dd2014 val2 = 0x55ac76dd2014
8) C Functions MCQs
115. what is a function in c.
- User defined data type
- Block of code which can be reused
- Declaration syntax
B) Block of code which can be reused
Function in C is a block of code which can be reused.
116. Functions in C can accept multiple parameters. True or False?
Functions in C programming can accept multiple parameters.
117. Which keyword is used to return values from function?
- Return type
C) Return type
The return in declaration is initialized using return type.
118. Which of these is not a valid parameter passing method in C?
- Call by value
- Call by reference
- Call by pointer
C) Call by pointer
Valid parameter passing method in C is:
119. A C program contains ___.
- At least one function
- No function
- No value from command line
A) At least one function
The main() function is required in the C program.
120. A recursive function in C ___.
- Call itself again and again
- Loop over a parameter
- Return multiple values
A) Call itself again and again
Recursive function in C is a function which calls itself again and again.
121. The scope of a function is limited to?
- Current block
- Only function
C) Whole file
The scope of a function is limited to the file it is declared in.
122. Which of the below syntax is the correct way of declaring a function?
- return function_name () { }
- data_type function_name (parameter) { }
- Void function_name ( )
The correct syntax of declaring a function in C:
123. The sqrt() function is used to calculate which value?
- Square of reverse bits
- Square root
C) Square root
The sqrt() method in C is used to calculate the square root of a number.
124. What will be the output of the following C program?
9) c pointers mcqs, 125. before using a pointer variable, it should be ___..
- Initialized
- Both A. and B.
C) Both A. and B.
A pointer variable should be declared and initialized before the using.
126. An uninitialized pointer in C is called ___.
- Void pointer
- Empty pointer
- Invalid pointer
- Wild pointer
D) Wild pointer
An uninitialized pointer in C is called wild pointer. Since, the pointer is initialized it may lead a program to behave wrongly or to crash.
127. ___ is a pointer that occurs at the time when the object is de-allocated from memory without modifying the value of the pointer.
- Dangling pointer
- Null pointer
A) Dangling pointer
A dangling pointer is a pointer that occurs at the time when the object is de-allocated from memory without modifying the value of the pointer.
128. A ___ can be assigned the address of any data type.
C) Void pointer
A void pointer can be assigned the address of any data type.
129. Which pointer is called general-purpose pointer?
A void pointer is called general-purpose, because it can be used with any type of variable.
130. Pointer arithmetic is not possible on ___.
- Integer pointers
- Float pointers
- Character pointers
- Void pointers
D) Void pointers
Pointer arithmetic is not possible on void pointers due to lack of concrete value and size.
131. Comment on the following pointer declaration?
- p is a pointer to integer, x is not
- p and x, both are pointers to integer
- p is pointer to integer, x may or may not be
- p and x both are not pointers to integer
A) p is a pointer to integer, x is not
In the above given statement, variable p is a pointer to integer, while x is an integer variable.
132. What will be the output of the following C code?
- A garbage value
In the above program, x contains 10 and ptr is a pointer to x . We are changing the value of x with the help of pointer. Thus, the value will be changed and it will be 20.
133. What will be the output of the following C code?
- IncludeHelp
- Some address
In the above program, in printf statement – we are using two symbols those are * and &. The * is a dereference operator, and the & is a reference operator. These symbols can be used any number of times. Here ptr points to the first character in the string "IncludeHelp". *ptr dereferences it and so its value is I. Again & references it to an address and * dereferences it to the value I.
134. What is the correct syntax to declare pointer to pointer i.e., double pointer?
- type **pointer_name;
- type *&pointer_name;
- type *(*pointer_name);
- type **(pointer_name);
A) type **pointer_name;
The correct syntax syntax to declare pointer to pointer i.e., double pointer is:
135. What is ptr in the given statement?
- ptr is an array of 5 pointers
- ptr is a simple integer array
- ptr is a pointer to a 5 elements integer array
C) ptr is a pointer to a 5 elements integer array
In the above statement, ptr is a pointer to a 5 elements integer array.
136. What is the correct syntax to declare a pointer to a constant?
- const type *pointer_name;
- type const *pointer_name;
A) const type *pointer_name;
The correct syntax to declare a pointer to a constant is:
10) C File Handling MCQs
137. which function is used to open a file in c.
- file_open()
In C programming language, the fopen() function is used to open a file.
Where, fileopen is the name of the file, and mode is the mode of the file.
138. Which character(s) is/are used to open a binary file in append mode in C?
The characters "ab" opens a binary file in append mode.
139. Which character(s) is/are used to open a binary file in reading and writing mode in C?
The characters "rb+" opens a binary file in reading and writing mode.
140. Which is the correct syntax to declare a file pointer in C?
- File *file_pointer;
- FILE *file_pointer;
- File file_pointer;
B) FILE *file_pointer;
The correct syntax to declare a file pointer in C is:
141. Which function is used to close an opened file in C?
- file_close()
- fileclose()
B) fclose()
In C programming language, the fclose() function is used to close an opened file.
142. Function fwrite() works with ___.
- Binary files
B) Binary files
Function fwrite() works with binary files. The file should be opened/created in binary mode.
143. What is the value of EOF in C?
The EOF stands for End of File which is a Macro defined in stdio.h header file. With the GNU C Library, EOF is -1. In other libraries, its value may be some other negative number.
144. Which function checks the end-of-file indicator for the given stream in C?
The C library function feof() checks the end-of-file indicator for the given stream.
145. Which function is used to seek the file pointer position in C?
In C programming language, the fseek() function is used to seek the file pointer position.
- file_pointer− This is the pointer to a FILE object that identifies the stream.
- offset − This is the number of bytes to offset from whence.
- whence − This is the position from where offset is added, the value of this parameter may SEEK_SET, SEEK_CUR, or SEEK_END.
146. Which function is used to delete an existing file in C?
D) remove()
In C programming language, the remove() function is used to delete an existing file.
Where, filename is the name of the file to be removed.
11) C Preprocessor MCQs
147. what is cpp in c programming.
- C processing platform
- C PreProcessor
- C pre-platform
B) C PreProcessor
In C programming, C preprocessors are referred to as CPP.
148. Which symbol is used to begin a preprocessor?
All preprocessor commands begin with a hash symbol (#).
149. Which of these is a valid preprocessor in C?
150. (\) operator in c is ___..
- Macro continuation operator
- Stringize operator
A) Macro continuation operator
Macro continuation (/) operator is used to continue a macro which is too long for a single line.
151. Which operator is used to stringize variables in C?
# Operator is used to stringize variables in C .
152. What will be the output of the following C code?
- Learn python programming language at includehelp
- Learn #a programming language at #b
- Learn programming language at
A) Learn python programming language at includehelp
153. Is it possible in a C program to merge two tokens into one token?
The ### preprocessor in C is used to merge two tokens to one.
154. Which of these is not a valid preprocessor in C?
\ and ### are both valid preprocessors in C.
155. Header files ___.
- Contain function declarations
- Can be included to a program
- End with .h extension
All statements are correct. The header files are special files included in a program that contain functions and macros which can be imported and used in the code. The extension of header files is ".h".
156. Can programmers create their own header files?
A header file can be created by a programmer with some function that she needs to use in other files as well.
157. Which of these is valid syntax to include a header in C?
- #include <header>
- #include "header"
C) Both A and B
The valid syntaxes to include a header file to a program in C are:
158. What will happen if a header file is included in a program twice?
- Program will throw an exception
- Program will run normally
A) Program will throw an error
When the same header is included twice in the program, the compiler will throw an error as its teens to process it twice.
159. Which syntax is correct to include a specific preprocessor based on configuration?
- #defif system1 <system.h>
- #include system1 "system.h"
- import <system.h> if system1
B) #include system1 "system.h"
The correct way to include a specific preprocessor based on configuration is known as computed include is:
Comments and Discussions!
Load comments ↻
- Blockchain MCQs
- Artificial Intelligence MCQs
- Data Analytics & Visualization MCQs
- Python MCQs
- C++ Programs
- Python Programs
- Java Programs
- D.S. Programs
- Golang Programs
- C# Programs
- JavaScript Examples
- jQuery Examples
- CSS Examples
- C++ Tutorial
- Python Tutorial
- ML/AI Tutorial
- MIS Tutorial
- Software Engineering Tutorial
- Scala Tutorial
- Privacy policy
- Certificates
- Content Writers of the Month
Copyright © 2024 www.includehelp.com. All rights reserved.
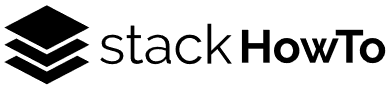
- 100 Multiple Choice Questions In C Programming – Part 1
T his collection of 100 Multiple Choice Questions and Answers (MCQs) In C Programming : Quizzes & Practice Tests with Answer focuses on “C Programming”.
1. A preprocessor directive is a statement that begins with_____
- Incorporation of source files (#include),
- Definition of symbolic constants and macros (#define),
- Conditional compilation (#if, #ifdef,…).
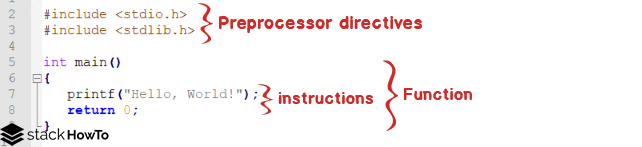
2. Which character always ends a statement?
3. how to write a comment on a single line.
A /* my comment
B // my comment //
C // my comment
D /* my comment */
If your comment is long, type your comment between (/* and */). For example:
4. What is a library?
A A source file already written containing ready-made functions
B A file allowing to display text on the screen
C A file containing my program
D None of the above
5. What is the name of the main function of a C program?
A principal
6. Which of the following functions display “Hello, World!” on the screen in console mode?
A echo("Hello, World!");
B print("Hello, World!");
C printf("Hello, World!");
D show("Hello, World!");
7. Which of the following characters is used to make a line break on the screen?
8. which of the following characters returns the cursor to the left of the screen, 9. what kind of files can be created with c programming.
A Images (*.jpg, *.png, *.gif…)
B Executables (*.exe on Windows)
C Sources (*.c)
D Text files (*.txt)
10. The program that translates your code from a high-level language to the binary language is called ________
A programmer
C translator
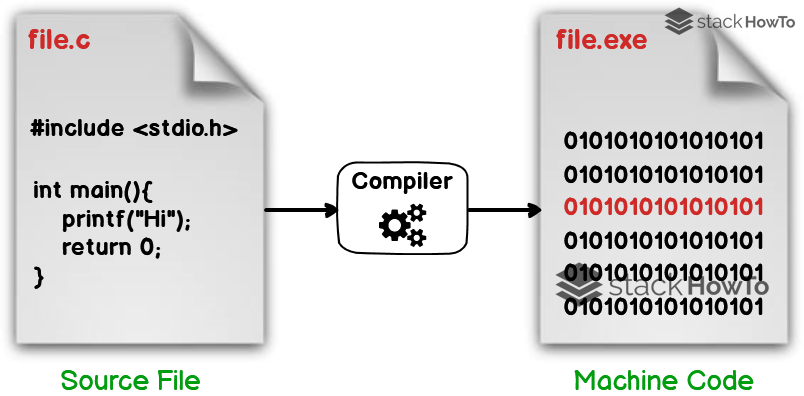
100 Multiple Choice Questions In C Programming – Part 2
- 100 Multiple Choice Questions In C Programming – Part 3
- 100 Multiple Choice Questions In C Programming – Part 4
- 100 Multiple Choice Questions In C Programming – Part 5
100 Multiple Choice Questions In C Programming – Part 6
100 multiple choice questions in c programming – part 7.
- 100 Multiple Choice Questions In C Programming – Part 8
- 100 Multiple Choice Questions In C Programming – Part 9
- 100 Multiple Choice Questions In C Programming – Part 10
- 100 Multiple Choice Questions In C Programming – Part 11
- 100 Multiple Choice Questions In C Programming – Part 12
- 100 Multiple Choice Questions In C Programming – Part 13
- 100 Multiple Choice Questions In C Programming – Part 14
- HTML/CSS MCQs – Multiple Choice Questions and Answers – Part 5
You May Also Like
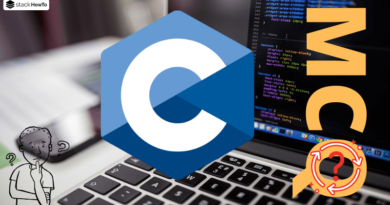
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.

- C Programming Tutorial
- Basics of C
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Type Casting
- C - Booleans
- Constants and Literals in C
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- Operators in C
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- Decision Making in C
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- Functions in C
- C - Functions
- C - Main Function
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- Scope Rules in C
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- Arrays in C
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- Pointers in C
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- Strings in C
- C - Strings
- C - Array of Strings
- C - Special Characters
- C Structures and Unions
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Structure Padding and Packing
- C - Nested Structures
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- File Handling in C
- C - Input & Output
- C - File I/O (File Handling)
- C Preprocessors
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Header Files
- Memory Management in C
- C - Memory Management
- C - Memory Address
- C - Storage Classes
- Miscellaneous Topics
- C - Error Handling
- C - Variable Arguments
- C - Command Execution
- C - Math Functions
- C - Static Keyword
- C - Random Number Generation
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Cheat Sheet
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework . You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
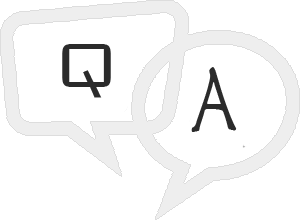
Q 1 - What is the output of the below code snippet?
C - Compile error
Explanation
2.30, addition is valid and after decimal with is specified for 2 places.
Q 2 - The type name/reserved word ‘short’ is ___
A - short long
B - short char
C - short float
D - short int
short is used as an alternative of short int.
Q 3 - Special symbol permitted with in the identifier name.
The only permitted special symbol is under score (_) in the identifier.
Q 4 - What is the output of the following program?
*a refers to 10 and adding a 1 to it gives 11.
Q 5 - What is the output of the following program?
D - Runtime error
Loop continues until *s not equal to ‘\0’, hence printing “Abc” where character is fetched first and address is incremented later.
Q 6 - In Windows & Linux, how many bytes exist for near, far and huge pointers?
A - Near: 1, far: 4, huge: 7
B - near: 4, far: 4, huge: 4
C - near: 0, far: 4, huge: 4
D - near: 4, far: 5, huge: 6
In DOS, numbers of byte exist for near pointer = 2, far pointer = 4 and huge pointer = 4.
In Windows and Linux, numbers of byte exist for near pointer = 4, far pointer = 4 and huge pointer = 4.
Q 7 - A bitwise operator “&” can turn-off a particular bit into a number.
B - &&
The bitwise AND operator “&” compares each bit of the first operand with the corresponding bit of the second operand. During comparison, if both operands bits are 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
Q 8 - How many times the given below program will print "IndiaPIN"?
A - Unlimited times
B - 0 times
C - 100 times
D - Till stack run over
A stack over flow comes when over loaded memory is used by the call stack. Here, main()function is called repeatedly and its return address stores in the stack. When stack memory get filled, it displays the error “stack overflow”.
Q 9 - Which of the following statement shows the correct implementation of nested conditional operation by finding greatest number out of three numbers?
A - max = a>b ? a>c?a:c:b>c?b:c
B - a=b ? c=30;
C - a>b : c=30 : c=40;
D - return (a>b)?(a:b) ?a:c:b
Syntax is exp1?exp2:exp3. Any ‘exp’ can be a valid expression.
Q 10 - In the given below code, what will be the value of a variable x?
C - Print x
D - Return Error
Although, integer y = 100; and constant integer x is equal to y. here in the given above program we have to print the x value, so that it will be 100.

IMAGES
VIDEO
COMMENTS
50 C Language MCQs with Answers Quiz will help you to test and validate your C Quiz knowledge. It covers a variety of questions, from basic to advanced. The quiz contains 50 questions.
This C Multiple Choice Questions article will cover all the basic to advanced MCQs on C programming language which are selected under the guidance of the masters of C and by getting ideas through the experience of students' recent C programming MCQ Test.
1000+ Multiple Choice Questions on C Programming arranged chapterwise! Start practicing C MCQ now for exams, online tests, quizzes, and interviews! C Language MCQ PDF covers topics like C Data Types, Pointers, Arrays, Functions, String Operations, Structures, Input & Output, C Preprocessor, etc.
Master C Programming with Practice MCQs. Explore our curated collection of Multiple Choice Questions. Ideal for placement and interview preparation, our questions range from basic to advanced, ensuring comprehensive coverage of C Programming.
This C Exercise page contains the top 30 C exercise questions with solutions that are designed for both beginners and advanced programmers. It covers all major concepts like arrays, pointers, for-loop, and many more.
100+ C Language Solved MCQs These multiple-choice questions (MCQs) are designed to enhance your knowledge and understanding in the following areas: Bachelor of Business Administration in Computer Applications (BBA [CA]) . Chapters
Practice problems on arrays using C. Improve your C programming skills with over 200 coding practice problems. Solve these beginner friendly problems online to get better at C language.
C language MCQs: This section contains multiple-choice questions and answers on the C programming language. It will help the students to test their skills and prepare well for their exams. List of C programming MCQs. C Basics MCQs; C Data Types, Operators and Expressions MCQs; C Conditional Statements MCQs; C Control Statements MCQs; C Strings MCQs
T his collection of 100 Multiple Choice Questions and Answers (MCQs) In C Programming : Quizzes & Practice Tests with Answer focuses on “C Programming”. 1. A preprocessor directive is a statement that begins with_____ The preprocessor is a program executed during the first stage of compilation.
C Programming - Online Quiz - Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer.